How to determine check/uncheck checkboxes in tree in angular? Mastering interactive tree structures in Angular applications often hinges on effectively managing checkboxes. This guide delves into the intricacies of handling checkboxes within Angular tree components, from basic implementation to advanced scenarios involving large datasets and complex interactions.
We’ll explore various methods for controlling checkbox states, examining both two-way data binding and custom events. Furthermore, this comprehensive walkthrough addresses data structures, best practices, and error handling, ensuring a robust and scalable solution for your Angular projects.
Introduction to Angular Tree Components
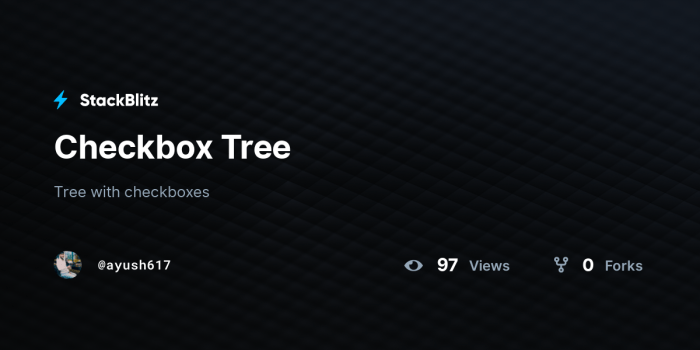
Angular tree components are crucial for displaying hierarchical data structures in web applications. They allow developers to represent complex relationships visually and interactively. This is particularly useful in scenarios like file systems, organizational charts, and nested categories. Their dynamic nature enables efficient data management and user interaction with the hierarchical information.Tree components in Angular are powerful tools for structuring and presenting data.
They are designed to handle large datasets and complex relationships effectively. They improve user experience by making data navigation intuitive and allowing for a better understanding of the information presented.
Types of Tree Structures
Different tree structures cater to diverse data representation needs. The most common types include:
- Hierarchical Trees: These trees represent data in a parent-child relationship, where each node can have multiple children. This structure is commonly used for displaying nested categories or organizational charts.
- Multi-select Trees: These structures allow users to select multiple nodes from the tree, often with the ability to select entire branches or specific nodes. They are important for applications requiring complex selections of data points.
- Virtualized Trees: These are designed to handle large datasets by loading only the visible portions of the tree. This is crucial for preventing performance bottlenecks when dealing with extensive hierarchical data.
Importance of Handling Checkboxes in Tree Structures
Checkboxes in tree components allow for selective data selection. This is valuable in applications needing to choose specific items from a hierarchy. For instance, in a file explorer, users can select specific files or folders. This ability is essential in applications that require dynamic filtering or data manipulation based on user selections. Furthermore, checkboxes enable users to efficiently filter, sort, and process the data represented in the tree structure.
Example of a Tree Component with Checkboxes
Imagine a tree component displaying product categories. Each category node has a checkbox, allowing users to select specific categories for further processing. This is a fundamental part of e-commerce applications where users can filter products by category.
// Component code snippet (illustrative)
// ... imports ...
class MyTreeComponent
treeData: any[] = [
id: 1, name: 'Electronics', children: [], checked: false ,
id: 2, name: 'Clothing', children: [], checked: false ,
id: 3, name: 'Books', children: [], checked: false
];
// ... methods for handling checkbox selection, e.g., toggleCheck(node) ...
This example shows a simplified structure. A real-world implementation would involve more sophisticated data structures and methods for handling checkboxes, updating the model, and potentially handling nested levels within the tree. A robust Angular tree component would also include features for managing the state of the checked nodes, enabling efficient filtering and data retrieval based on user selections.
Methods for Handling Checkboxes: How To Determine Check/uncheck Checkboxes In Tree In Angular
Understanding how to manage checkboxes within an Angular tree structure is crucial for building interactive and user-friendly applications. Efficiently controlling checkbox states, particularly within complex hierarchical structures, requires careful consideration of data binding and event handling. This section will explore various methods for managing checkboxes in Angular trees, emphasizing the balance between simplicity and scalability.
Controlling checkbox states within an Angular tree involves understanding how changes in one checkbox can cascade through the tree structure. This requires meticulous management of parent-child relationships, and a thorough understanding of data flow within the application. This section will illustrate best practices for handling checkboxes in an Angular tree, including techniques for managing large tree structures.
Two-Way Data Binding
Two-way data binding offers a straightforward approach for synchronizing checkbox states with the application’s data model. This approach directly connects the checkbox’s value to a property within the component’s class. Changes in the checkbox’s state automatically update the corresponding property, and vice versa. This simplicity is attractive for smaller, less complex tree structures.
Custom Events
Custom events offer a more flexible alternative to two-way data binding, particularly in more intricate tree structures. By emitting custom events when a checkbox changes state, the component can explicitly manage the update process. This approach allows for finer control over how checkbox toggles propagate through the tree structure. This method can be advantageous when complex logic or validation needs to be applied to the checkbox updates.
Updating Parent/Child Relationships
Updating parent/child relationships when checkboxes are toggled requires careful consideration of the tree structure. When a checkbox is checked or unchecked, the corresponding parent and child elements must be updated to reflect the change. This typically involves iterating through the tree structure and updating the relevant properties accordingly. Consider using recursive functions for traversing the tree to maintain consistency.
Managing Large Tree Structures
Managing large tree structures with checkboxes demands strategies for efficiency. Avoid unnecessary recalculations and updates. Consider using techniques like memoization or caching to optimize the update process. As the tree grows, the performance impact of update operations will become more significant. Using appropriate data structures and optimizing algorithms are vital to prevent performance degradation.
Reactive Forms for Checkbox States
Reactive forms provide a structured approach for managing checkbox states within an Angular application. They allow for declarative configuration of form controls, including checkboxes, and provide validation and control capabilities. This approach offers advantages when dealing with complex forms or intricate interactions. Reactive forms are useful when managing checkbox states in a structured manner within a larger application.
Consider using reactive forms to handle form validation and complex logic surrounding checkbox interactions.
Implementing Checkbox Functionality
Implementing checkbox functionality in an Angular tree component requires careful consideration of how to manage the checked state of individual checkboxes and how those changes propagate through the tree structure. This section details the implementation, tracking mechanisms, and potential challenges. A well-designed system will ensure accurate and reliable data representation.
Checkbox State Tracking
The core of checkbox functionality is tracking the checked state of each checkbox. This is often achieved using a data model that mirrors the tree structure. Each node in the data model would contain a property indicating its checked status. This property could be a simple boolean value (true for checked, false for unchecked). The data model should be structured to allow for easy access and manipulation of individual node checkboxes.
Propagation of Changes
Changes in checkbox states must be propagated accurately to parent and child nodes. When a checkbox is checked or unchecked, the corresponding change in the data model should trigger updates in the UI. This requires event handling and appropriate data binding in Angular. Careful attention to event triggers is critical to maintain consistency between the data model and the UI representation.
A well-designed system should avoid unintended consequences when a checkbox in the tree is toggled.
Handling Large Numbers of Checkboxes
Implementing checkboxes in a tree with a large number of nodes can present performance challenges. The UI may become sluggish if updates are not optimized. Techniques like using change detection strategies and optimizing data binding are crucial to handle large data sets. Careful consideration of the data structure, data binding, and event handling is necessary to avoid performance degradation.
For example, if the tree is extremely deep and has thousands of nodes, batch updates can be a better strategy than triggering updates for each node individually. Consider using `ChangeDetectionStrategy.OnPush` to prevent unnecessary updates.
Multiple Checkbox Selection
Handling multiple checkbox selections requires the ability to collect and manage the selected nodes. This can be achieved by maintaining an array of selected node IDs or objects. This array should be updated whenever a checkbox state changes. The array will represent the selection and should be readily accessible for downstream operations. For example, if a user selects multiple nodes in a tree, a list of the selected items can be generated and displayed elsewhere in the application.
It’s essential to provide mechanisms for clearing the selection if needed.
Example
Consider a scenario where a user can select nodes in a file system-like tree. The data model might represent each file/folder as a node with a `checked` property. A service could be used to collect and manage the `checked` nodes, providing methods to get all selected items and clear the selection. The UI can update the selected nodes list in real-time.
Data Structures and Interactions
Understanding the proper data structure for a tree component with checkboxes is crucial for maintaining a clear and consistent representation of the data. This structure should facilitate easy manipulation and updates to the checked states of checkboxes, particularly within nested hierarchies. Proper data organization will also ensure that changes in one part of the tree automatically reflect in other parts, maintaining data integrity.
Data Structure for Nested Checkboxes
The data structure should reflect the hierarchical nature of the tree. Each node in the tree should represent an item and include properties to manage its state and the state of its children. A crucial aspect of this structure is how the checked state of each node is represented.
- A suitable data structure for a tree with checkboxes is an array of objects. Each object represents a node in the tree. The object will contain properties such as the node’s value, its parent node (if any), and a boolean property to indicate whether the checkbox is checked.
- The `children` property is vital for representing nested checkboxes. This property holds an array of child objects, enabling the recursive representation of the tree structure.
- For example, a node might have properties like `id`, `text`, `checked`, and `children`. The `children` property would hold an array of objects, each representing a child node. This recursive structure allows for arbitrary nesting depth.
Example Data Structures
Illustrative examples demonstrate various data structures suitable for nested checkboxes.
-
Consider a simple tree structure. Each node has an `id`, `text`, `checked` property, and a `children` array. The example below illustrates the structure, representing a parent node and its children:
[ id: 1, text: 'Parent 1', checked: false, children: [ id: 2, text: 'Child 1', checked: false, children: [] , id: 3, text: 'Child 2', checked: true, children: [] ], id: 4, text: 'Parent 2', checked: true, children: [] ]
This structure directly reflects the hierarchy and allows for easy access to child nodes.
Representing Checked State
The `checked` property, a boolean value, directly indicates the checked status of the corresponding checkbox. If `checked` is `true`, the checkbox is checked; otherwise, it is unchecked. This straightforward representation is crucial for efficient management of checkbox states.
Parent-Child Interactions
Changes in the checked state of a parent node should cascade down to its children, and vice versa. Similarly, changes in the checked state of a child should update the parent.
- When a parent checkbox is checked, all its children should be checked, and when unchecked, all its children should be unchecked. Conversely, when a child checkbox is checked, its parent should be updated to reflect that change.
- To achieve this, an update function can be used. This function will iterate through the children of the modified node and change their `checked` status accordingly.
Handling State Changes Affecting Other Parts
The data structure should be designed to accommodate the impact of checkbox state changes on other parts of the application.
- For example, if a checkbox represents a selection for a particular item, the application logic should reflect the selected items. This could involve filtering data, updating a display, or performing a calculation.
- This interaction with other parts of the application is crucial for the overall functionality of the system.
Best Practices and Considerations
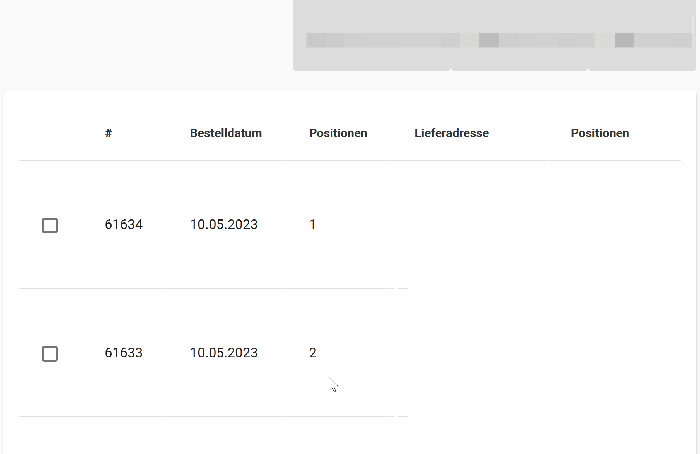
Implementing checkboxes within Angular tree components requires careful consideration of various factors. Proper implementation ensures a robust and user-friendly experience, especially when dealing with extensive data. Best practices, along with performance and scalability strategies, are crucial for a smooth user interface.
Thorough planning and implementation are essential to avoid common pitfalls when managing complex tree structures. This involves meticulous consideration of data structures, asynchronous operations, and error prevention. Understanding the nuances of Angular services and their role in data management further enhances the quality of the application.
Best Practices for Implementing Checkboxes
Implementing checkboxes in Angular tree components requires adherence to best practices for maintainability and scalability. Consistency in design and implementation choices is vital for creating a user-friendly interface. Utilizing Angular’s built-in features and established design patterns is recommended to ensure a robust and reliable user experience. Maintain clear naming conventions for variables and components to enhance code readability.
Use meaningful names that reflect the purpose of each component or variable, aiding in future maintenance and collaboration.
Performance and Scalability Considerations
When dealing with a large number of checkboxes in an Angular tree component, performance and scalability become critical. Inefficient data handling can lead to significant performance bottlenecks. Using efficient data structures and optimized algorithms is vital for a smooth user experience. Avoid unnecessary data retrieval or manipulation to prevent slowdowns. Implement lazy loading techniques to load data only when needed.
This significantly reduces initial load times and improves overall performance, particularly when handling extensive data.
Preventing Errors in Updating Tree Structure
Careful handling of updates to the tree structure is essential when checkboxes are modified. Implementing robust validation and error-handling mechanisms is crucial. Use immutable data structures to avoid unintended side effects. Implementing a clear strategy for data validation and error handling helps prevent inconsistencies and ensures data integrity. Thorough testing of the component with various scenarios is necessary to identify and address potential issues.
Using Angular Services for Managing Checkbox Data
Angular services offer a structured approach to managing data related to checkboxes. Services provide a centralized location for data access and modification, reducing redundancy and promoting code organization. Separate the logic for handling checkbox state changes from the component’s view logic. Maintain consistency in data format and structure across the application. This promotes maintainability and facilitates collaboration among team members.
Handling Asynchronous Operations
Asynchronous operations, such as fetching data from an API, are common when dealing with checkboxes in Angular tree components. Implementing asynchronous operations requires careful consideration to avoid blocking the UI thread. Use observables to manage asynchronous data streams. Employ techniques to manage potential errors during asynchronous operations and provide appropriate feedback to the user. Display loading indicators while data is being fetched to keep the user informed about the application’s status.
Implementing error handling in the asynchronous operations prevents the application from crashing or displaying unexpected results to the user.
Handling Selection and Deselection
Properly handling checkbox selection and deselection in a tree structure is crucial for maintaining data integrity and user experience. This involves understanding how changes in one part of the tree might affect other parts and how to manage these interactions effectively. Careful consideration of selection and deselection logic ensures that the application behaves predictably and reliably.
Selecting or deselecting a checkbox in a tree structure often triggers cascading effects on other checkboxes within the same tree or related components. A key aspect is understanding the impact of these actions on the underlying data model and how the application updates in response. This requires careful design of the data structures and the logic that governs selection and deselection.
Managing Checkbox Selection
Selection of checkboxes in a tree structure involves updating the application’s data model to reflect the user’s choices. This often involves recursively traversing the tree structure, updating the selected states of all child checkboxes and the parent checkboxes accordingly. Maintaining consistency between the UI and the data model is critical to prevent unexpected behavior.
Implementing Selection Logic
A well-structured approach to managing selection involves creating a method that updates the selected state of a checkbox. This method should take the checkbox’s state (selected or deselected) and its corresponding data as input. The logic should propagate the change to related checkboxes and update the associated data accordingly.
Handling Subtree Selection
When a checkbox in a subtree is selected or deselected, the selection state of its parent and child checkboxes needs to be updated automatically. This requires careful implementation of the data model and the logic for propagating the change. Consider how the change affects other parts of the application. For instance, if a specific checkbox’s selection triggers a calculation, the calculation should be updated accordingly.
Using ngModel for Checkbox Management
Using Angular’s `ngModel` for managing checkboxes in a tree structure provides a straightforward way to bind the checkbox’s selected state to the application’s data model. The `ngModel` directive simplifies the binding process, allowing the application to update the data model and the UI automatically.
“`javascript
// Example using ngModel
node.name
“`
Custom Controls for Checkbox Handling
Custom controls offer greater flexibility in managing checkboxes, allowing for complex selection logic and interaction with other parts of the application. These custom controls can be designed to handle specific requirements or provide more granular control over selection.
Impact on Other Components
Selection changes in the tree might trigger actions or updates in other components of the application. This requires careful consideration of how the tree’s selection impacts other data and components. For example, if selecting a checkbox in the tree triggers a calculation in another component, the logic for handling this interaction should be clearly defined. This might involve event emitters, services, or other communication mechanisms to update the other components accordingly.
Error Handling and Validation
Ensuring the integrity and accuracy of user input is crucial in any application, especially when dealing with selections like checkboxes within a tree structure. Robust error handling and validation prevent unexpected behavior, maintain data consistency, and safeguard against potential issues stemming from user interaction. This section will Artikel best practices for managing errors and validating checkbox selections within Angular tree components.
Potential Errors During Checkbox Interactions
Incorrect data formatting, missing data, or user input mistakes can lead to errors during checkbox interactions. These errors can manifest as unexpected behavior, data inconsistencies, or even application crashes. Carefully identifying and handling these errors is essential for a smooth user experience.
Validation Rules for Checkbox Selections
Validation rules dictate acceptable checkbox selection patterns. These rules, defined according to the application’s logic and requirements, prevent invalid or illogical selections. For example, a rule might enforce that at least one checkbox within a specific group must be selected. Defining these rules beforehand is vital for maintaining data integrity and user experience.
Examples of Validating User Input
To illustrate, consider an application where users select project tasks within a hierarchical tree structure. A validation rule might require that a project has at least one task selected, and that tasks are selected in a hierarchical manner. This can be achieved by using Angular’s reactive forms or custom validation functions. For instance, using a reactive form, a custom validator can be created to ensure the parent checkboxes are selected before child checkboxes.
Scenario for Demonstrating Validation Rules
Imagine a project management application. Users can select tasks within a project hierarchy. A validation rule could ensure that:
- At least one task is selected under each project.
- If a parent task is selected, all child tasks must also be selected (or vice versa, if a child task is selected, the parent task must also be selected).
This scenario emphasizes the need for validation rules that reflect the application’s specific requirements.
Types of Validation Errors
Several types of validation errors can occur when dealing with checkbox selections:
- Empty Selections: A user might not select any checkboxes in a required field. This error necessitates a prompt to the user to select at least one option.
- Inconsistent Selections: A user might select a child checkbox without selecting its parent. This error should be highlighted, prompting the user to select the parent checkbox.
- Data Type Errors: Input data might not match the expected data type (e.g., selecting text instead of a boolean value). A clear error message should guide the user to correct the input.
- Range Errors: Selections might exceed the allowable range. This could occur if a user is only permitted to select a certain number of checkboxes.
- Logical Errors: Selections might violate the application’s logic. For example, a user may select mutually exclusive options, violating the application’s rules. These errors need to be handled with clear messages guiding the user to valid selections.
Displaying Results and State
Presenting the results of checkbox selections in a clear and user-friendly manner is crucial for a good user experience. A well-designed display allows users to quickly understand the choices they’ve made and their impact. This section details how to effectively display the selected items and their corresponding information.
Displaying the Current State of Selected Checkboxes
The core of displaying selection results is updating the UI to reflect the current state of selected checkboxes. This involves retrieving the selected items from the data model and then presenting them in a structured way. The data structure needs to be accessed and iterated upon to extract and display relevant data.
Presenting Selected Items in a Formatted Way, How to determine check/uncheck checkboxes in tree in angular
A simple list might not be sufficient for complex data. A more organized format, like a table, is often preferable. This allows for better visualization of the selected items and their attributes. A table layout facilitates the presentation of various data attributes.
Visualizing Results in a User-Friendly Manner
A user-friendly display should be clear, concise, and easy to navigate. Consider using appropriate colors, fonts, and spacing to enhance readability. A well-organized table is an effective way to visualize the selected items, enabling users to scan and grasp the information quickly.
Designing a User Interface for Displaying Selected Items
The UI for displaying selected items should align with the overall design of the application. A consistent layout, along with appropriate styling, ensures a smooth user experience. The design must prioritize clear presentation of data and intuitive interaction with the selected items.
Organizing a Table Displaying Selected Items
A table structure is suitable for displaying multiple attributes of the selected items. A table facilitates clear visualization of various data points. Use HTML table tags for structuring the table.
Item Name | Category | Description | Status |
---|---|---|---|
Item A | Category X | Detailed description of Item A. | Selected |
Item B | Category Y | Detailed description of Item B. | Selected |
Item C | Category Z | Detailed description of Item C. | Not Selected |
This table provides a clear view of selected items, their categories, descriptions, and status. The responsiveness of the table design should be considered for different screen sizes. The table’s structure should align with the underlying data structure.
Final Conclusion
In conclusion, handling checkboxes within Angular tree components requires a well-structured approach, encompassing data organization, interactive elements, and careful consideration for performance. This guide has provided a roadmap for implementing and maintaining robust tree structures with checkboxes, enabling seamless user interactions and a polished user experience.
Questions Often Asked
How do I efficiently manage a large tree structure with checkboxes?
Employing optimized data structures and techniques for updating the tree incrementally can significantly improve performance. Consider using a virtual scrolling approach for handling large numbers of nodes, updating only the relevant parts of the UI.
What are some common pitfalls when handling checkbox interactions in large trees?
Performance issues, especially when dealing with numerous checkboxes and complex interactions, can arise. Careful attention to data management and UI updates is crucial. Avoid redundant data manipulation and focus on efficient update strategies.
How do I validate user selections in the tree?
Implement validation rules to ensure the user’s selection meets specific criteria. These rules could involve minimum/maximum selection counts, restrictions on selecting specific types of nodes, or mandatory selections for certain branches.
How can I handle asynchronous operations when updating checkbox states?
Use promises or Observables to manage asynchronous operations effectively. This ensures that the UI updates correctly even when data loading or processing takes place. Employ appropriate error handling to address any issues during the asynchronous process.