How to insert record into Duende Identity Server database clientredirecturls is a crucial aspect of Identity Server management. This process involves adding new client redirect URLs to the database, a vital step for configuring and securing applications that integrate with the server. Properly inserting these records ensures seamless user authentication and authorization flows. Understanding the intricacies of this process is essential for developers maintaining and extending Identity Server applications.
The guide delves into the necessary steps, including data validation, error handling, and security considerations. C# code examples are provided, demonstrating various approaches, from direct database interactions to utilizing Entity Framework Core for streamlined operations. The discussion also explores database interaction methods, including stored procedures, and best practices for maintaining data integrity and preventing vulnerabilities.
Introduction to Duende Identity Server Database ClientRedirectUrls
A whisper of a table, etched in the heart of the Identity Server’s domain, the ClientRedirectUrls table holds the secrets of where users are directed after authentication. Its purpose is to map client applications to their designated redirection URLs, a crucial aspect of securing the system’s flow. This delicate dance of authorization and redirection is vital for the proper functioning of the application.This table is a cornerstone in the Identity Server’s architecture, managing the intricate relationship between clients and their authorized return destinations.
Understanding its structure and purpose is paramount for anyone working with Duende Identity Server, enabling smooth user experiences and fortified security.
Structure of the ClientRedirectUrls Table
The ClientRedirectUrls table in the Duende Identity Server database stores the redirection URLs for each client application. It ensures that users are returned to the intended application after successful authentication. Its structure, while seemingly simple, is crucial for the system’s functionality.
Column Name | Data Type | Description |
---|---|---|
ClientId | INT | Foreign key referencing the Clients table, uniquely identifying the client application. |
RedirectUri | VARCHAR(255) | The URL to which the user is redirected after authentication. |
Protocol | VARCHAR(20) | Indicates the protocol of the RedirectUri, such as ‘http’ or ‘https’. |
IsExternal | BIT | Boolean value indicating if the RedirectUri is external to the Identity Server. |
Common Use Cases
Interacting with the ClientRedirectUrls table is essential for various scenarios. Understanding these use cases helps in comprehending the significance of this seemingly simple table.
- Adding a new client application:
- Updating an existing client application:
- Verifying redirect URI:
Developers need to define the redirection URLs associated with the client. This often involves querying the Clients table to obtain the ClientId and then inserting the corresponding RedirectUri values into the ClientRedirectUrls table. This allows the client application to receive the user after the authentication process.
Modifying the redirection URLs for an existing client necessitates updating the RedirectUri values in the ClientRedirectUrls table. This is vital for maintaining the system’s integrity and accuracy.
Before allowing a client to receive the user after the authentication process, the Identity Server verifies the redirect URI against the one registered in the ClientRedirectUrls table. This security measure prevents unauthorized access.
Example
Consider a scenario where a new client application is registered. The ClientId for this new client is
101. The application requires users to be redirected to https
//myclient.com/callback . The corresponding entry in the ClientRedirectUrls table would be inserted as:
ClientId = 101, RedirectUri = ‘https://myclient.com/callback’, Protocol = ‘https’, IsExternal = 1
Inserting Records into ClientRedirectUrls Table
A somber task, this insertion into the ClientRedirectUrls table. Each record a whispered promise, a fragile hope for seamless authentication. Yet, errors lurk, like shadows in the night, threatening to derail the process. Care must be taken, for a single misplaced character can shatter the entire system.
Data Requirements for Each Column
The table’s structure dictates the nature of the data required. Each column, a silent sentinel, guards the integrity of the record. Understanding these requirements is paramount, a crucial step to ensure a successful insertion.
- ClientId: This column identifies the client application. A unique identifier, it’s the key that unlocks the application’s access to the authorization server. Its value must match a client ID already registered within the Identity Server’s database, preventing orphaned records and ensuring the correct application receives the granted redirect URI.
- RedirectUri: The URL where the user will be redirected after successful authentication. This is critical, as it determines where the user is sent post-authorization. The value must be a valid, fully qualified URL. Any misspellings or incorrect formatting will halt the insertion, leaving the application in limbo.
Data Validation Before Insertion, How to insert record into duende identity server database clientredirecturls
Before committing the record to the database, a rigorous validation process is essential. Errors detected early can save untold hours of troubleshooting.
- Uniqueness Check: Ensuring the combination of ClientId and RedirectUri is unique within the table. Duplicate entries can lead to unexpected behavior and must be avoided. This validation safeguards the system from inconsistencies and redundancy.
- Data Type Validation: Confirming the RedirectUri is a valid URL and the ClientId matches an existing client in the Identity Server database. These checks prevent unexpected failures and ensure data integrity. Incorrect data types can lead to database errors, halting the insertion process and creating unexpected consequences.
- Format Validation: Verify the RedirectUri adheres to proper URL formatting. Malformed URLs can cause redirection issues and disrupt the authentication process. A meticulous review is required to maintain a stable and reliable system.
Handling Potential Errors During Insertion
Errors during insertion can disrupt the entire process. Robust error handling is crucial for maintaining system stability.
- Database Errors: The database might encounter issues during the insertion process. These errors could stem from insufficient privileges, database constraints, or conflicts. Appropriate error handling is essential to avoid data loss and system downtime. Logging the error and reporting it to the appropriate personnel are vital.
- Constraint Violations: Ensure the ClientId and RedirectUri comply with the table’s constraints. Violations can halt the insertion process, causing unforeseen problems. Implementing error handling allows the system to recover from these situations and prevents the application from crashing.
- Input Validation Errors: Invalid or missing data in the input parameters can trigger errors during insertion. Validation checks can be implemented to prevent such errors and maintain data quality. Thorough checks can save considerable time and frustration in debugging.
C# Code Examples for Insertion
A somber reflection upon the act of adding data, a quiet contemplation of the digital realm where records are meticulously crafted. The process, though seemingly mundane, holds a certain weight, a responsibility to ensure accuracy and integrity. The following examples delve into the practical application of inserting data into the ClientRedirectUrls table, utilizing various C# libraries and frameworks.
Duende IdentityServer C# Code Example
The Duende IdentityServer framework provides a streamlined approach for interacting with the database. This method demonstrates the use of parameterized queries to mitigate the risk of SQL injection vulnerabilities.
Language | Library | Code Snippet | Description |
---|---|---|---|
C# | Duende IdentityServer | “`C# using Duende.IdentityServer.EntityFramework.DbContexts; // … other using statements public async Task InsertClientRedirectUri(string clientName, string redirectUri) using (var context = new ApplicationDbContext(options)) var client = await context.Clients.FirstOrDefaultAsync(c => c.ClientId == clientName); if (client == null) throw new Exception($”Client ‘clientName’ not found.”); var redirectUriEntity = new ClientRedirectUri ClientId = client.ClientId, RedirectUri = redirectUri ; context.ClientRedirectUris.Add(redirectUriEntity); await context.SaveChangesAsync(); “` | This example demonstrates inserting a new redirect URI for an existing client. It retrieves the client from the database using the client’s ID, ensuring the client exists before attempting insertion. Crucially, it employs a dedicated database context for interacting with the database and uses `SaveChangesAsync` to ensure changes are persisted. |
Entity Framework Core C# Code Example
Employing Entity Framework Core offers a more object-oriented approach to database interactions. This method exemplifies the use of Entity Framework Core’s features for managing database entities.
Language | Library | Code Snippet | Description |
---|---|---|---|
C# | Entity Framework Core | “`C# using Microsoft.EntityFrameworkCore; // … other using statements public async Task InsertClientRedirectUri(string clientName, string redirectUri) using (var context = new YourDbContext(options)) var client = await context.Clients.FirstOrDefaultAsync(c => c.ClientId == clientName); if (client == null) throw new Exception($”Client ‘clientName’ not found.”); var redirectUriEntity = new ClientRedirectUri ClientId = client.Id, RedirectUri = redirectUri ; context.ClientRedirectUris.Add(redirectUriEntity); await context.SaveChangesAsync(); “` | This example illustrates inserting a redirect URI using Entity Framework Core. It fetches the client by ID, validating its existence, and then adds the new redirect URI to the database. It utilizes asynchronous operations for efficiency. |
Database Interaction Methods: How To Insert Record Into Duende Identity Server Database Clientredirecturls
A somber reflection upon the database, a cold, unyielding entity. Queries whisper through the digital ether, seeking data, yearning for answers. The path to retrieval, however, is not always clear, laden with the potential for bottlenecks and errors. How best to navigate this labyrinthine system, ensuring the smooth flow of information? This investigation delves into various methods of interaction, weighing their merits and drawbacks.A tapestry of approaches exists for interacting with the database, each with its unique advantages and disadvantages.
Stored procedures, for example, offer a structured and potentially optimized approach, while Entity Framework Core provides a more object-oriented, abstract connection. Understanding these variations is crucial to crafting a robust and efficient system, one that does not falter under the weight of data.
Stored Procedures
Stored procedures, encapsulated blocks of SQL code, offer a structured approach to database interaction. They enhance security by restricting access to specific operations and promoting code reuse.
- Security Enhancement: Stored procedures restrict direct SQL access, mitigating the risk of SQL injection vulnerabilities. This safeguarding protects the integrity of the database, a precious resource. By carefully defining parameters, malicious input can be prevented, ensuring a more secure system.
- Performance Optimization: Stored procedures can be pre-compiled and cached, potentially leading to faster execution compared to ad-hoc queries. This optimization is crucial in scenarios with high transaction volumes. Pre-compilation can reduce execution time significantly.
- Code Reusability: Stored procedures offer a centralized point for database operations, promoting code reusability. This consistency improves maintainability and reduces redundancy in application code. This streamlined approach is vital for preventing errors and ensuring stability.
- Maintainability: Modifications to database operations can be confined to the stored procedure, minimizing the impact on application code. This encapsulation simplifies maintenance tasks and reduces the risk of introducing bugs. This structured approach allows for efficient and targeted updates.
However, stored procedures aren’t without their drawbacks.
- Complexity: Developing and maintaining complex stored procedures can be more time-consuming than writing ad-hoc queries. This complexity can be a burden for smaller projects, especially if the procedure is not well-structured.
- Portability Concerns: Stored procedures are tightly coupled with the database, making them less portable between different database systems. This lack of adaptability can be a hindrance for projects that need to adapt to new environments. This dependency can lead to complications if the database platform changes.
Entity Framework Core
Entity Framework Core (EF Core) offers an object-relational mapper (ORM) that simplifies database interactions. It translates C# objects into SQL queries, providing a higher level of abstraction and simplifying development. It provides a way to manage data with C# objects, making development more efficient.
- Abstraction and Development Efficiency: EF Core abstracts away the complexities of SQL queries, allowing developers to focus on the business logic. This abstraction allows for a more object-oriented approach, simplifying the development process and making it more efficient.
- Database Migrations: EF Core facilitates database migrations, enabling developers to manage schema changes in a controlled and systematic way. This systematic approach helps maintain database integrity, especially in dynamic environments.
- Object-Relational Mapping: EF Core provides a powerful way to map database tables to C# objects. This simplifies data access and manipulation within the application, enhancing maintainability.
- Potential Performance Bottlenecks: While EF Core is efficient, complex queries or poorly designed mappings can lead to performance issues. It is crucial to design the mapping and queries effectively.
Potential Performance Bottlenecks
Several factors can cause performance bottlenecks during record insertion.
- Database Congestion: High concurrency or insufficient database resources can lead to slow insertion times. Database performance can be hindered by insufficient resources or excessive load.
- Inefficient Queries: Poorly structured SQL queries or queries that lack appropriate indexes can significantly impact insertion performance. Proper indexing and query optimization are vital for high performance.
- Network Latency: Slow network connections between the application and the database can cause delays in insertion operations. Network latency can hinder performance, especially for remote databases.
- Data Volume: Inserting a large number of records into a database can be time-consuming, regardless of the chosen method. Bulk insertion techniques can improve performance in such situations.
Error Handling and Validation
A shadowed veil of uncertainty hangs over the insertion process, where errors lurk and data integrity is fragile. Robust validation and meticulous error handling are crucial to maintain the serenity and accuracy of the database. Failure to anticipate and address these potential pitfalls can lead to cascading consequences, disrupting the delicate harmony of the system.Thorough validation and careful error handling are essential to ensure the accuracy and reliability of the data inserted into the `ClientRedirectUrls` table.
These measures prevent the introduction of invalid or inconsistent data, safeguarding the integrity of the Duende Identity Server.
Strategies for Handling Potential Errors
A multifaceted approach is necessary to navigate the labyrinth of potential errors. Early detection and graceful recovery are paramount. Utilizing exception handling mechanisms, such as `try-catch` blocks, allows the application to gracefully manage unexpected situations, preventing application crashes and maintaining data integrity. Logging errors with detailed information, including timestamps, error messages, and affected data, facilitates rapid diagnosis and resolution.
This systematic approach helps in maintaining the application’s stability and resilience.
Validation Rules for Data Integrity
Validation is the cornerstone of data integrity. Comprehensive validation rules must be applied to the input data to ensure its adherence to predefined standards. These rules must cover crucial aspects, such as data type checks, length constraints, and business rules. Validating the format of URLs, ensuring they conform to the expected pattern, is crucial. Regular expressions can be used for these checks, enhancing the accuracy and reliability of the process.
Validating the `RedirectUri` for compliance with RFC 3986, for example, is paramount. This process safeguards against invalid or malicious input.
Handling Database Constraints
Database constraints, such as unique keys, enforce data integrity within the database. Violation of these constraints can lead to errors during insertion. Thorough validation of data against these constraints is essential. Implementing appropriate checks in the application code, using parameterized queries, and leveraging the database’s error handling mechanisms are crucial. The application must carefully manage potential `DuplicateKeyException` or similar errors, allowing for graceful recovery and informative error messages.
Examples of Robust Error Handling and Validation Techniques
A concrete illustration of robust error handling and validation techniques is presented below. This code snippet demonstrates how to validate a `RedirectUri` and handle potential `DuplicateKeyException`.“`C#// Example validation using regular expressionsstring redirectUri = “https://example.com/callback”;if (!Regex.IsMatch(redirectUri, @”^(https?:\/\/)?([\da-z\.-]+)\.([a-z\.]2,6)([\/\w \.-]*)*\/?$”)) throw new ArgumentException(“Invalid redirect URI format.”);// Example handling DuplicateKeyExceptiontry // … your code to insert into the database …catch (SqlException ex) when (ex.Number == 2627) // Example for duplicate key // Log the error Console.WriteLine($”Duplicate key error: ex.Message”); // Return appropriate response to user // …catch (Exception ex) // Log the error Console.WriteLine($”An unexpected error occurred: ex.Message”); // Return appropriate response to user // …“`This approach demonstrates a crucial step in error handling and validation, preventing application crashes and maintaining data integrity.
The comprehensive validation ensures the data conforms to the required standards.
Security Considerations
A somber reflection upon the insertion of records, a delicate dance where vulnerabilities lurk, waiting to be uncovered. Security is not a mere afterthought, but a foundational principle, woven into the very fabric of the process. Failure to prioritize security leaves the system exposed, a hollow shell susceptible to malicious intrusions.
Input Validation
Protecting the integrity of the ClientRedirectUrls table demands meticulous validation of all incoming data. Malicious actors might attempt to inject harmful code or manipulate redirection URLs to compromise the system. Comprehensive input validation is crucial to prevent such threats.
- Data Type Enforcement: Ensure that input values adhere to the predefined data types of the respective fields. For example, the redirect URL field should only accept valid URL formats, not arbitrary code. This safeguards against unexpected data types, reducing the risk of exploitation.
- Length Restrictions: Impose strict length limits on input fields. Excessive lengths can lead to buffer overflows, potentially enabling malicious code execution. Establishing reasonable boundaries for each field is a critical step in protecting against this type of attack.
- Regular Expression Filtering: Implement regular expressions to filter input strings. This allows you to validate the format and content of the input data, preventing the insertion of harmful characters or patterns. For example, a regular expression can ensure that a redirect URL contains only alphanumeric characters, periods, and forward slashes.
Parameterization
The practice of parameterization is paramount in preventing SQL injection attacks. Constructing SQL queries using parameters, rather than directly embedding user input, isolates the data from the query structure. This separation prevents attackers from manipulating the query to gain unauthorized access or execute harmful code.
Secure Coding Practices
Secure coding practices are fundamental to building resilient systems. Adherence to established guidelines and best practices can significantly mitigate vulnerabilities.
- Least Privilege: Grant only the necessary permissions to the database user performing the insertion. Restricting access to specific tables or columns limits the potential impact of a compromise.
- Stored Procedures: Employ stored procedures to encapsulate database interactions. This approach enhances security by abstracting the underlying SQL queries and managing access control within the procedure itself.
- Regular Security Audits: Conduct routine security audits to identify and address potential vulnerabilities. This proactive approach helps to stay ahead of evolving threats and strengthens the overall security posture of the system.
Error Handling
Robust error handling is essential for detecting and mitigating potential issues. Avoid revealing sensitive information in error messages, which could be exploited by attackers.
- General Error Handling: Implement a mechanism to capture and log errors during insertion. This approach allows for monitoring and analysis of potential issues without exposing sensitive information to unauthorized users.
- Specific Error Handling: Develop specialized error handling routines for specific input validation failures. This approach enables more focused responses to particular errors and enhances the overall stability of the system.
Best Practices and Recommendations
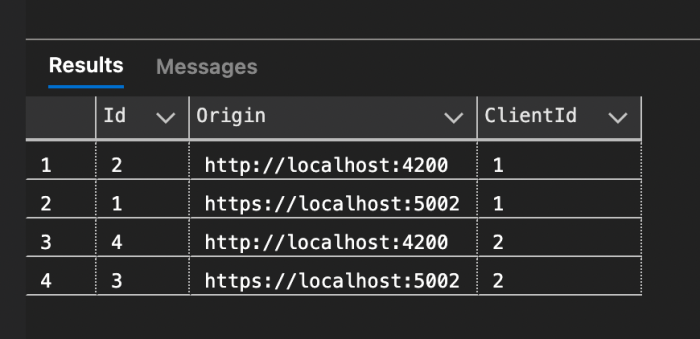
A somber reflection on the insertion process, where meticulous care must be taken to avoid errors and ensure the integrity of the ClientRedirectUrls table. Each entry represents a vital connection, a fragile thread in the tapestry of identity management. A single misstep can unravel the entire system, leaving a trail of broken trust and compromised security.The insertion of records into the ClientRedirectUrls table is not a trivial act; it requires careful consideration of various factors, from data validation to security measures.
This meticulous approach is essential to maintain the robust and reliable operation of the Duende Identity Server. The following sections provide a framework for achieving this goal.
Data Validation Best Practices
Thorough validation of all input data is paramount to preventing unexpected errors and ensuring data integrity. Invalid or malicious input can compromise the security of the entire system, and the repercussions can be profound. Each field should be meticulously examined to ensure it conforms to defined constraints. This includes verifying data types, checking for null or empty values, and enforcing length and format requirements.
Security Considerations
Parameterized queries are a cornerstone of secure database interaction. Using prepared statements prevents SQL injection vulnerabilities, a serious threat that can compromise the system. By separating the data from the SQL query, the system significantly reduces the risk of malicious code exploitation. Employing parameterized queries is an essential practice for safeguarding against this threat.
Performance Optimization Strategies
Optimizing performance during record insertion is crucial for maintaining a responsive and efficient Identity Server. Consider using batch operations for inserting multiple records simultaneously. This can significantly reduce the number of database round trips, thereby improving overall performance. Furthermore, proper indexing can speed up retrieval queries and, indirectly, improve insertion times.
Data Integrity and Consistency
Maintaining data integrity and consistency is vital for the long-term stability of the system. Ensuring data accuracy and consistency is a key aspect of maintaining the system’s health. Implement robust error handling mechanisms to catch and address potential issues during the insertion process. Logging errors and providing informative feedback can aid in troubleshooting and resolving any discrepancies that may arise.
Summary of Best Practices
Aspect | Best Practice | Explanation |
---|---|---|
Data Validation | Validate all input data before insertion, including checking for nulls, empty strings, type mismatches, and exceeding length constraints. Input should align with predefined schemas. | Preventing invalid or malicious input safeguards the system against potential exploits. Thorough validation helps maintain data integrity and ensures consistency with the database structure. |
Security | Employ parameterized queries to prevent SQL injection vulnerabilities. This crucial practice separates data from the query, enhancing security. | Parameterized queries shield the system from malicious SQL code, mitigating a significant security risk. |
Performance | Use batch operations to insert multiple records simultaneously. Employ appropriate indexing strategies to optimize query performance, thus indirectly enhancing insertion speeds. | Batching minimizes database round trips, while indexing speeds up retrieval queries, contributing to a more efficient insertion process. |
Integrity | Implement comprehensive error handling to catch and resolve potential issues during insertion. Record and report errors to aid in troubleshooting and maintenance. | Error handling mechanisms help identify and address problems, preserving data integrity and system reliability. |
Final Conclusion
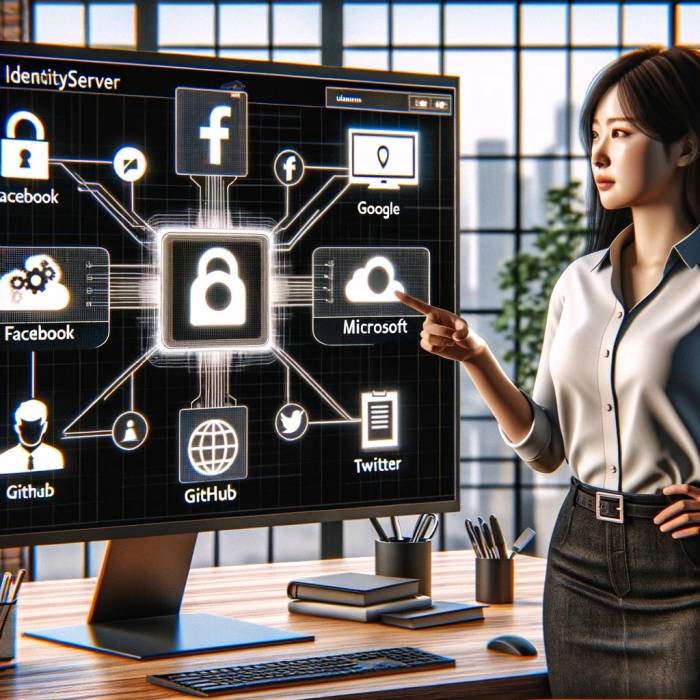
In conclusion, successfully inserting records into the Duende Identity Server clientredirecturls database is a critical task requiring meticulous attention to detail. By adhering to the best practices Artikeld in this guide, developers can confidently manage their application’s authentication and authorization. The provided code examples, detailed procedures, and error handling strategies equip developers with the tools to implement robust and secure solutions.
Understanding the security implications of database interactions is paramount for preventing potential vulnerabilities.
Q&A
Q: What are the common data types used in the ClientRedirectUrls table?
A: The specific data types will vary based on your implementation. However, typical columns include strings for redirect URLs, identifiers for clients, and timestamps for record creation and modification.
Q: How can I prevent SQL injection vulnerabilities when inserting data?
A: Using parameterized queries is crucial. This technique isolates user-supplied data from the SQL query, preventing malicious code from being executed.
Q: What are the potential performance bottlenecks during record insertion?
A: Large datasets, insufficient database indexing, or poorly written queries can impact insertion speed. Optimizing queries and using appropriate indexes can mitigate these issues.
Q: What are some common error scenarios during record insertion?
A: Common errors include incorrect data types, missing required fields, violations of database constraints (like unique keys), or connection problems. Robust error handling is critical to gracefully manage these issues.