How to build a calculator interview question? This intricate query probes not just your coding prowess, but your understanding of algorithms, data structures, and even user experience. It’s a multifaceted challenge, demanding a nuanced approach from basic arithmetic to advanced scientific functions. The question delves into the entire process, from conceptual design to practical implementation and rigorous testing.
Preparing for this interview question involves a deep understanding of the various aspects of calculator design. From the logical flow of operations to the user interface, and from error handling to advanced features, each component plays a crucial role in building a robust and efficient calculator. This detailed guide will equip you with the knowledge and strategies to excel in this important interview.
Understanding the Interview Question
The interview question “how to build a calculator” is deceptively simple. It’s not about merely constructing a basic addition tool. Instead, it probes your understanding of fundamental programming principles, algorithm design, and data structures. A strong response will demonstrate not only the ability to code but also the thought process behind creating a robust and potentially scalable solution.The question encompasses a wide range of interpretations, from basic arithmetic operations to sophisticated scientific calculations, potentially including handling user input, error conditions, and even memory management.
The depth and breadth of the response expected directly correlate with the level of the position being interviewed for.
Different Interpretations of the Question
The “calculator” can be interpreted at various levels of complexity. A basic calculator might only handle simple arithmetic operations (addition, subtraction, multiplication, division). A more advanced calculator could include trigonometric functions, exponential functions, logarithms, and potentially even support complex numbers. The scope of the project depends heavily on the interviewer’s expectations. The interviewer might want to assess your ability to handle a wide variety of inputs or to perform complex calculations with precision.
Levels of Complexity
The complexity of the expected response can vary significantly. A junior-level candidate might be asked to implement basic arithmetic operations, focusing on fundamental programming constructs. Senior-level candidates, on the other hand, might be challenged to design a calculator with error handling, user-friendly interfaces, and potentially even memory management. The interviewer will often look for considerations beyond just functional correctness, such as efficiency and robustness.
Underlying Concepts
The design of a calculator involves several crucial programming and algorithm concepts. These include:
- Input Handling: Parsing user input to extract numbers and operators, validating input for correctness (e.g., preventing division by zero). Handling different input formats, like scientific notation or parentheses.
- Operator Precedence: Implementing correct order of operations (e.g., multiplication before addition). Data structures like stacks or recursion can be employed for evaluating expressions according to precedence rules.
- Algorithm Design: Selecting the appropriate algorithm for evaluating the expression (e.g., the Shunting-Yard algorithm). The choice of algorithm affects efficiency and correctness. Consider the scalability and maintainability of the chosen algorithm.
- Error Handling: Designing mechanisms to handle potential errors (e.g., division by zero, invalid input). Providing meaningful error messages is critical for user experience.
Logical Structure of a Calculator
A calculator’s functionality can be broken down into a logical structure, including:
- Input Module: This module receives user input, be it numbers, operators, or functions. Input validation is essential to prevent unexpected behavior or crashes. Examples include checking for invalid characters or attempting to divide by zero.
- Processing Module: This module performs the necessary calculations. It must adhere to the order of operations and handle various mathematical functions, possibly involving complex logic and data structures to ensure accurate results.
- Output Module: This module displays the results of the calculations. The output needs to be formatted in a user-friendly manner, considering different formats, like scientific notation or decimal places, for different types of calculations. This module is also responsible for handling and displaying potential error messages.
Designing the Calculator’s Logic
A fundamental aspect of calculator design involves creating the core logic that performs arithmetic operations. This section delves into the algorithms and data structures employed for accurate and efficient calculation. Understanding potential errors, particularly division by zero, is crucial for robust calculator functionality. Different approaches to implementing the calculator’s logic are also discussed, considering the trade-offs between efficiency and readability.The calculator’s logic forms the heart of its operation.
It defines how the calculator interprets user input, executes calculations, and presents the results. Designing robust and efficient algorithms for addition, subtraction, multiplication, and division, along with error handling for exceptional cases like division by zero, is critical for a reliable calculator application.
Basic Arithmetic Operations
The core arithmetic operations—addition, subtraction, multiplication, and division—are implemented using straightforward algorithms. These algorithms are crucial for the calculator’s fundamental functionality.
- Addition: The addition operation involves summing two numbers. The algorithm simply adds the corresponding digits of the two numbers, carrying over any excess values to the next significant digit. For example, to add 123 and 456, the algorithm aligns the numbers vertically, adds the digits in the ones place (3 + 6 = 9), the tens place (2 + 5 = 7), and the hundreds place (1 + 4 = 5).
The result is 579.
- Subtraction: Subtraction is the inverse of addition. The algorithm involves subtracting the corresponding digits of the two numbers, borrowing from higher significant digits when necessary. For instance, to subtract 456 from 789, the algorithm aligns the numbers, subtracts the digits in the ones place (9 – 6 = 3), the tens place (8 – 5 = 3), and the hundreds place (7 – 4 = 3).
The result is 333.
- Multiplication: Multiplication involves repeated addition. The standard algorithm involves multiplying each digit of one number by each digit of the other number and then summing the partial products. For example, to multiply 12 by 34, we multiply 12 by 4 (48) and then 12 by 30 (360). The sum of these partial products (48 + 360) equals 408.
- Division: Division involves determining how many times one number (the divisor) is contained within another number (the dividend). The standard long division algorithm is used to determine the quotient and remainder. For example, dividing 128 by 4 involves determining how many times 4 goes into 12 (3 times) and then continuing with the algorithm. The result is 32 with a remainder of 0.
Error Handling: Division by Zero
Division by zero is a critical error condition that must be handled. This prevents the calculator from producing incorrect or undefined results.A robust calculator implementation includes a mechanism to detect and report division by zero errors. This is accomplished by checking if the divisor is zero before performing the division operation. If the divisor is zero, the calculator should display an appropriate error message, such as “Division by zero is not allowed.”
Data Structures for Complex Calculations
For more complex calculations, data structures like stacks or queues can enhance the calculator’s efficiency.
- Stacks: Stacks can be useful for evaluating expressions using the order of operations (e.g., parentheses). The stack data structure is particularly well-suited for managing operands and operators in an expression.
- Queues: Queues are useful for managing tasks in a specific order. In a calculator application, a queue could be used for processing multiple expressions or tasks sequentially.
Implementation Approaches
Various approaches can be used to implement the calculator’s logic.
- Using Functions: Defining separate functions for each arithmetic operation (addition, subtraction, etc.) provides modularity and readability. This approach enhances code organization and makes maintenance easier.
- Using Operators: Employing operators (e.g., +, -,
-, /) allows for concise expression evaluation. This method can lead to more compact code. Operator overloading is another option to support different data types.
Implementing the User Interface
A robust calculator extends beyond its core logic; a compelling user interface (UI) is crucial for usability and user satisfaction. The UI design dictates how users interact with the calculator and how the results are presented. A well-designed UI ensures a positive user experience, making the calculator intuitive and efficient to use.The UI implementation translates the design into a functional interface, incorporating input methods and display formats.
Careful consideration of these elements is paramount for a successful calculator application. This process involves mapping user actions to calculator operations, ensuring smooth data flow between input, processing, and output.
User Interface Design
The user interface design of a calculator needs to prioritize clarity and ease of use. Input methods should be straightforward, allowing users to enter numbers and operators with minimal effort. Display formats are critical, influencing how results are presented to the user.
Input Methods
The calculator’s input methods must be intuitive and easily accessible. A standard approach involves physical buttons, each representing a number, operator, or function. For modern applications, touchscreens allow for a more flexible input system, using virtual buttons or a text entry field. The method should be user-friendly and prevent errors during data entry. A combination of physical and virtual buttons can be employed for the best possible user experience.
Display Formats
Different display formats cater to various user needs and calculator functionalities.
- Simple Display: This format typically displays a single line of text, showing the current input and calculation results. Suitable for basic arithmetic calculations, it’s a common choice for handheld calculators. The display will typically show a single line of numbers and operators.
- Scientific Display: For advanced calculations, a scientific display is essential. It features more buttons for trigonometric, logarithmic, and exponential functions, along with more complex operators. The display should accommodate multiple lines or a larger area to display the formulas and results accurately. Examples include scientific notation and multiple lines for complex equations.
- Graphical Display: A graphical display offers a more visual representation of calculations, particularly useful for plotting functions and graphs. This approach can be particularly useful for engineering or scientific applications. The display would need to handle plotting and graphing capabilities. It’s an advanced format, requiring more complex UI components.
User Experience (UX) Considerations
User experience (UX) plays a significant role in the overall success of a calculator application. A positive UX ensures the calculator is easy to use and enjoyable to interact with. Key aspects of UX include:
- Intuitive Navigation: The UI should be organized in a logical and intuitive manner, allowing users to quickly find the functions they need.
- Clear Visual Hierarchy: Important elements should stand out visually, such as buttons for frequently used functions.
- Accessibility: The calculator should be accessible to users with disabilities, following accessibility guidelines for keyboard navigation and screen readers. This ensures that the calculator is usable by a wide range of users.
- Error Handling: A good UI provides clear feedback to the user in case of errors or invalid inputs, such as displaying an error message.
User Interface Principles
Following UI principles ensures a user-friendly design.
- Consistency: Maintain consistent design elements and layout throughout the calculator to provide a predictable user experience.
- Simplicity: Avoid unnecessary complexity. The UI should be straightforward and easy to learn.
- Feedback: Provide visual or auditory feedback to the user when they interact with the calculator.
- Readability: Use a clear font size and style to ensure the display is easy to read.
Handling Input and Output
Successfully constructing a calculator necessitates meticulous handling of user input and presentation of results. This involves translating user-provided data into a format suitable for calculations, and then effectively displaying the outcome in a user-friendly manner. Error handling is critical to maintain the calculator’s robustness and provide a positive user experience.
Input Handling
Input handling is pivotal for a functional calculator. Users interact with the calculator via buttons or text fields, and the system must accurately interpret this input. This involves converting textual input into numerical values.
- Parsing Input Strings: User input is typically in string format. A critical step is to parse these strings into numerical values. Consider using dedicated functions (e.g.,
parseFloat()
orparseInt()
in JavaScript) to handle various input formats (integers, decimals, scientific notation) and to isolate numerical components. Robust parsing is crucial for handling potentially complex user inputs. - Input Validation: Input validation is paramount for ensuring data integrity. Implement checks to ensure input values adhere to the calculator’s expected formats. This includes verifying if the input is a valid number (e.g., not containing letters or special characters) and ensuring it falls within acceptable ranges (if applicable). For example, checking for non-numeric input prevents errors during calculations.
- Input Buffering: For calculators with multi-step operations, consider implementing input buffering. This approach temporarily stores input data until the user presses an operator or an equals sign, preventing immediate evaluation and enabling users to correct mistakes before final calculation. This method is particularly useful in complex calculations.
Output Display
The output stage presents the results of calculations to the user. Clarity and accuracy are paramount.
- Result Display: The chosen output format should be intuitive. Displaying the result as a string is often sufficient for most cases, but for complex or scientific calculations, consider formatted output options. This includes formatting for decimals, exponents, or scientific notation.
- Clear Display: Ensure the display is clear and concise, with proper formatting for different numbers and operations. Consider using appropriate spacing and visual cues to separate different parts of the expression, if necessary.
- Error Handling in Output: Implement mechanisms to display meaningful error messages when calculations lead to errors, like division by zero or invalid operations. These messages should be user-friendly and informative. For instance, if the user attempts a division by zero, the calculator should output a clear message like “Division by zero is not allowed.”
Error Handling and Validation, How to build a calculator interview question
Error handling is a critical aspect of a robust calculator application. Invalid input can lead to unexpected results or crashes. Thorough error handling prevents these issues and enhances the user experience.
- Input Validation Techniques: Employ techniques like regular expressions to validate input data, checking for valid numerical formats and acceptable ranges. This validation should be performed before any calculation is attempted. For instance, a regular expression can ensure the input string adheres to a decimal format.
- Error Handling Strategies: Employ a comprehensive approach to handling potential errors. Use `try…catch` blocks to manage exceptions, such as invalid input or division by zero. Display user-friendly error messages when errors occur. These messages should be specific and informative to assist users in correcting the input.
Efficient Input/Output Management
Efficient management of input and output is crucial for a responsive calculator. Minimizing processing time ensures a smooth user experience.
- Input Optimization: Optimize the parsing of input strings to minimize processing time. Using optimized libraries or algorithms for string manipulation can improve performance. For example, using a lexer to break down complex input strings into individual tokens can streamline the input processing.
- Output Optimization: Optimize the formatting of output to reduce processing time. Using efficient string formatting techniques, such as string concatenation or string interpolation, can speed up the display of results.
Advanced Calculator Features
A scientific calculator extends beyond basic arithmetic to encompass a wider range of mathematical operations. This necessitates a design that prioritizes clarity, accuracy, and efficiency. The implementation of these features must consider potential user needs and the trade-offs between complexity and usability.
Trigonometric Calculations
Scientific calculators must support trigonometric functions (sine, cosine, tangent, and their inverse functions). These functions are crucial for solving problems in various fields, including engineering, physics, and mathematics. Implementing these functions requires libraries or custom algorithms that handle the calculation of trigonometric values accurately. This usually involves utilizing approximation methods or pre-computed tables to obtain values for the trigonometric functions.
The calculator should also allow the user to specify the angle unit (degrees or radians).
Memory Functions
Memory functions provide a way to store intermediate results and recall them later. This feature improves efficiency and allows users to perform complex calculations without losing intermediate results. A calculator should offer at least a memory recall function and memory storage functions, plus possibly a memory clear function. The memory should be designed to handle potential overflow situations, and it is important to define clear procedures for managing memory values (e.g., overwriting or clearing).
For example, a user might calculate the area of a circle and store the radius in memory, then use that stored value to calculate the circumference.
Advanced Operations
Logarithms and exponentiation are fundamental operations in scientific calculations. Logarithms (base 10 and natural log) are used to simplify complex calculations, and exponentiation is essential for calculations involving exponents and powers. The calculator must accurately handle different bases and input values. A calculator should offer the capability for calculations involving natural logarithms, base-10 logarithms, and exponents.
Implementation of Advanced Functions
The implementation of advanced functions involves choosing the right algorithms and data structures. For trigonometric functions, libraries often provide highly optimized implementations. For logarithms and exponentials, numerical methods are typically employed to approximate the results. Efficient algorithms are crucial for avoiding slowdowns in the user interface and ensuring accuracy. Using existing libraries can save significant development time and ensure correctness.
This can involve libraries optimized for numerical computation, ensuring the results meet expected accuracy and performance standards.
Trade-offs Between Simplicity and Functionality
A trade-off exists between adding advanced features and maintaining a user-friendly interface. Overloading the calculator with too many features can make it complex and difficult to use. A balanced approach is crucial to ensure that the calculator is accessible to users of all skill levels. Clear and intuitive menus, well-placed function buttons, and proper documentation are crucial for user experience.
It’s essential to prioritize the most commonly used features and provide additional features as optional or easily accessible secondary options.
Testing and Debugging
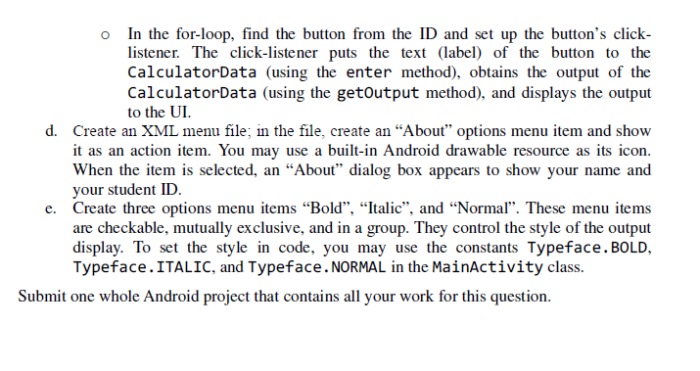
Thorough testing and debugging are crucial for ensuring the calculator’s accuracy, reliability, and robustness. A well-tested calculator will handle various inputs, edge cases, and potential errors gracefully, providing a positive user experience. This section details strategies for comprehensive testing and debugging, emphasizing the importance of anticipating and addressing potential issues.
Testing Strategies
Effective testing involves verifying the calculator’s functionality across a range of inputs and scenarios. This process ensures the calculator accurately performs the intended calculations and handles various input types correctly. Testing should cover both expected and unexpected inputs, including boundary conditions, to identify and mitigate potential errors.
- Unit Testing: Individual components of the calculator, such as functions for addition, subtraction, multiplication, and division, should be tested independently. This isolates potential problems and facilitates rapid identification and resolution of errors.
- Integration Testing: This strategy assesses the interaction between different modules of the calculator. It validates that the components work seamlessly together, ensuring the entire system operates as intended.
- System Testing: This is the most comprehensive approach. It tests the entire calculator system, simulating real-world usage scenarios. This ensures the calculator performs as expected under different conditions and load.
Test Cases
A comprehensive test suite should include various test cases to cover diverse scenarios. These test cases must validate the calculator’s accuracy and reliability across different inputs and operations.
- Basic Calculations: Test cases should include simple arithmetic operations like addition, subtraction, multiplication, and division with positive and negative numbers.
- Complex Calculations: Test cases should also involve complex calculations that involve multiple operations, ensuring the calculator handles order of operations correctly. Example: 10 + 5
– 2 – 3 / 2. - Edge Cases: Edge cases, such as division by zero, very large numbers, very small numbers, and invalid inputs, are crucial to test. The calculator should gracefully handle these cases without crashing or producing incorrect results.
- Boundary Conditions: Test cases should cover input values at the upper and lower limits of the calculator’s range, such as the maximum and minimum possible numbers the calculator can handle.
- Special Cases: Special cases like percentages, square roots, exponents, and trigonometric functions must also be included in the test suite.
Bug Identification and Resolution
Debugging involves systematically identifying and fixing errors in the calculator’s code.
- Debugging Tools: Employ debugging tools like print statements, breakpoints, and debuggers to step through the code, identify the source of errors, and understand the program’s flow during execution.
- Error Handling: Implement robust error handling mechanisms to catch and gracefully handle exceptions. For example, if a user enters an invalid input, the program should display an appropriate error message rather than crashing.
- Code Reviews: Conduct code reviews to identify potential issues, improve code quality, and ensure adherence to coding standards.
Comprehensive Testing Best Practices
Implementing comprehensive testing involves following established best practices to ensure the quality and reliability of the calculator.
- Test-Driven Development (TDD): Writing test cases before writing the actual code helps ensure that the code meets the requirements and functions correctly.
- Automated Testing: Automated testing frameworks can be employed to execute test cases repeatedly and consistently, ensuring high coverage.
- Code Coverage: Tools that measure code coverage can be used to ensure that the tests effectively cover the different parts of the code.
Handling Edge Cases
Edge cases represent unusual or unexpected inputs that might not be common in typical usage but can still cause errors. These need special consideration during testing to ensure the calculator’s robustness.
- Input Validation: Validate user inputs to prevent errors from unexpected data. Ensure inputs conform to expected formats, data types, and ranges.
- Error Handling: Implement error-handling mechanisms that catch and manage unexpected conditions or errors that might occur during calculation or input. This helps prevent the calculator from crashing or producing incorrect results.
- Comprehensive Test Cases: Design comprehensive test cases that include edge cases to thoroughly test the calculator’s behavior under various unusual conditions.
Illustrative Examples
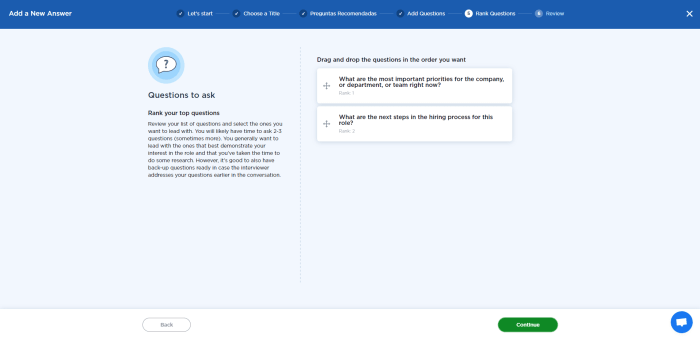
Demonstrating calculator implementations through various examples provides valuable insights into the design considerations and challenges involved. These examples showcase different levels of complexity, from a basic arithmetic calculator to a more advanced scientific calculator and even graphical user interfaces. Understanding these examples clarifies the process of building a robust and user-friendly calculator application.
Simple Calculator Implementation (Python)
A basic calculator in Python can be constructed using functions for each operation. This example focuses on the fundamental arithmetic operations.“`pythondef add(x, y): return x + ydef subtract(x, y): return x – ydef multiply(x, y): return x – ydef divide(x, y): if y == 0: return “Division by zero error” return x / ynum1 = float(input(“Enter first number: “))operator = input(“Enter operator (+, -,
, /)
“)num2 = float(input(“Enter second number: “))if operator == ‘+’: print(num1, “+”, num2, “=”, add(num1, num2))elif operator == ‘-‘: print(num1, “-“, num2, “=”, subtract(num1, num2))elif operator == ‘*’: print(num1, “*”, num2, “=”, multiply(num1, num2))elif operator == ‘/’: result = divide(num1, num2) if isinstance(result, str): print(result) else: print(num1, “/”, num2, “=”, result)else: print(“Invalid operator”)“`This code snippet demonstrates a simple calculator structure with functions for each operation.
It includes error handling for division by zero. Input validation is crucial for robustness.
Advanced Calculator Design (Scientific Calculator)
A scientific calculator extends beyond basic arithmetic to include trigonometric, logarithmic, exponential, and other scientific functions. A more sophisticated structure is needed.“`pythonimport math# … (Functions for basic operations from previous example) …def sin(x): return math.sin(x)def cos(x): return math.cos(x)# … (Add more scientific functions) …# … (Rest of the code for input and output similar to basic example) …“`This example highlights the expansion of functionality.
The inclusion of external libraries (like `math` in Python) is necessary to implement more complex calculations. A clear separation of concerns between the fundamental arithmetic and the more advanced scientific functions enhances code organization and readability.
Graphical Calculator Design
A graphical calculator provides a user-friendly interface. The UI can be created using libraries like Tkinter (Python) or similar tools in other languages.A graphical calculator typically employs a series of buttons for input, a display for output, and an event handling system to respond to user interactions.
Element | Description |
---|---|
Input Buttons | Buttons for numbers, operators, and functions. |
Display | Area to show input and output. |
Event Handling | Mechanism to respond to button presses and calculations. |
The layout and design choices are critical to ensure usability and aesthetics. Clear labeling and intuitive button placement enhance the user experience.
Error Handling in a Calculator
Robust error handling is crucial for a reliable calculator. This involves catching potential exceptions like division by zero, invalid input, or syntax errors.“`pythontry: result = divide(num1, num2) print(result)except ZeroDivisionError: print(“Division by zero error”)except ValueError as e: print(f”Invalid input: e”)“`This code snippet shows how to use `try-except` blocks to handle potential errors during calculations.
This prevents unexpected crashes and provides informative error messages to the user. Comprehensive error handling makes the calculator more user-friendly and resilient to unexpected inputs.
Structuring the Response for an Interview: How To Build A Calculator Interview Question
A well-structured response to an interview question about building a calculator demonstrates a candidate’s understanding of software design principles, algorithm selection, and practical implementation. This structured approach highlights the key elements of a robust and maintainable calculator application.A comprehensive response should cover various aspects of calculator design, from fundamental functionality to potential advanced features. It should exhibit a clear understanding of trade-offs in design choices, algorithm efficiency, and code maintainability.
Functionality, Algorithm, Implementation, and Testing
A structured approach to designing a calculator involves breaking down the problem into manageable components. This section Artikels a systematic way to approach the problem by focusing on the four crucial aspects: functionality, algorithm, implementation, and testing.
Functionality | Algorithm | Implementation | Testing |
---|---|---|---|
Basic arithmetic operations (addition, subtraction, multiplication, division) | Standard arithmetic algorithms | Implement functions for each operation using appropriate data types (e.g., floating-point numbers). | Test with various input values, including boundary cases (e.g., very large or very small numbers, zero division). |
Handling parentheses and order of operations | Shunting-yard algorithm or similar expression parsing algorithm. | Implement a parser to correctly evaluate expressions with parentheses and operator precedence. | Test with complex expressions to ensure correct evaluation of precedence and parentheses. |
Trigonometric functions (sin, cos, tan) | Implement using standard mathematical libraries or appropriate approximations. | Include these functions in the calculator’s functionality. | Test with various angles, including boundary cases (e.g., 0, 90, 180 degrees). |
Memory functions (e.g., storing and recalling values) | Implement memory storage and retrieval using a stack or similar data structure. | Add buttons or commands for memory functions to the user interface. | Test memory operations to ensure data is stored and retrieved correctly. |
Calculator Types and Features
Different types of calculators cater to varying needs. Understanding the types and features helps tailor the response to the specific calculator requested.
Calculator Type | Features |
---|---|
Basic Calculator | Basic arithmetic operations, percentage, memory functions. |
Scientific Calculator | Trigonometric functions, logarithmic functions, exponential functions, constants (Ď€, e), memory functions. |
Financial Calculator | Financial calculations (e.g., compound interest, amortization). |
Graphing Calculator | Graphing functions, numerical analysis, matrix operations. |
Programming Language Comparison
The choice of programming language significantly impacts development time and the calculator’s performance. Different languages offer varying advantages and disadvantages.
Programming Language | Advantages | Disadvantages |
---|---|---|
Python | Readability, extensive libraries (e.g., math, NumPy), rapid development. | Can be slower than compiled languages for computationally intensive tasks. |
Java | Platform independence, large community support, robust libraries. | Steeper learning curve for beginners, verbose syntax. |
C++ | High performance, control over memory management, fine-grained control. | Steeper learning curve, more complex to use. |
Structured Approach to Addressing the Interview Question
A structured response involves the following steps:
- Clearly define the scope of the calculator (e.g., basic, scientific). This ensures that the features and functionalities are well-defined.
- Detail the algorithm for each operation, focusing on efficiency and correctness. This showcases understanding of fundamental mathematical concepts and algorithm design.
- Explain the implementation choices, including data structures and libraries used. This highlights knowledge of programming techniques and software design patterns.
- Artikel the testing strategy, covering various cases to demonstrate the robustness of the calculator. This showcases attention to detail and the ability to identify potential issues.
Conclusion
Constructing a calculator, whether basic or advanced, reveals your programming abilities, problem-solving skills, and your keen eye for detail. This comprehensive exploration of the “how to build a calculator interview question” has covered every step from foundational logic to advanced features. Remember to structure your response clearly, emphasizing your understanding of algorithms, data structures, and user experience. The key is to demonstrate a systematic approach, from design to implementation, and showcase your ability to tackle real-world challenges with precision and efficiency.
FAQ Guide
What programming languages are suitable for implementing a calculator?
Python, JavaScript, Java, and C++ are all viable options, each with strengths in different areas. The best choice depends on the specific requirements of the calculator and your familiarity with the language.
How can I handle user input errors in the calculator?
Implement input validation to ensure the user enters valid numerical data. Provide informative error messages to guide the user and prevent unexpected behavior.
What are the key considerations for a scientific calculator?
Scientific calculators require support for trigonometric functions, logarithms, exponentials, and other advanced mathematical operations. Proper handling of these functions and their potential input variations is crucial.
How important is the user interface in a calculator design?
A user-friendly interface is vital for a successful calculator. Clear display, intuitive input methods, and well-placed controls contribute significantly to the overall user experience.