How to add back to top to blogspot blog? This guide walks you through various methods for adding a handy “Back to Top” button to your Blogger blog. We’ll cover everything from simple JavaScript implementations to more complex custom code solutions, ensuring your blog is user-friendly and visually appealing.
From customizing the button’s appearance to troubleshooting potential issues, this comprehensive tutorial will equip you with the knowledge and tools to create a seamless user experience. We’ll explore different styling options, animation techniques, and responsiveness considerations, ensuring your “Back to Top” button looks great on any device.
Methods for Implementing a “Back to Top” Button: How To Add Back To Top To Blogspot Blog
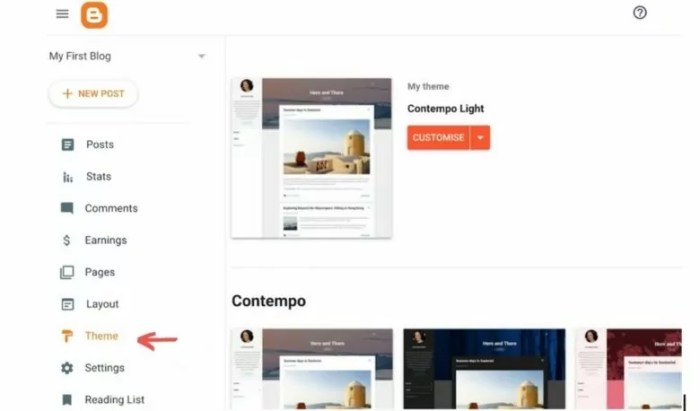
Adding a “Back to Top” button to your Blogger blog is like giving your readers a helpful little hand. It’s a simple feature that significantly improves user experience, making it easier for them to navigate your content. Imagine a long, sprawling blog post—without a “Back to Top” button, readers might get lost in the depths of your writing, losing their way and giving up on reaching the beginning.Implementing a “Back to Top” button is not rocket science, but it’s crucial to choose the right method for your blog.
The key is to find a balance between simplicity and effectiveness, especially if you want it to be visible and accessible for your readers.
Client-Side JavaScript Implementation
A client-side approach is often the easiest way to add a “Back to Top” button. It’s handled directly in the browser, meaning there’s no need for server-side modifications. This approach is ideal for quick fixes and allows for dynamic adjustments based on user scrolling.
- Utilizing JavaScript’s `window.onscroll` event: This is a powerful tool for tracking user scrolling. You can use it to dynamically show or hide the button based on how far down the page the user has scrolled. This ensures the button is only visible when needed, avoiding cluttering the screen. A good example would be when the user scrolls past a certain point on the page.
- Implementing a smooth animation: Instead of a sudden jump to the top, a smooth animation makes the experience more pleasant for the user. JavaScript offers numerous ways to achieve this effect, such as using CSS transitions or other animation libraries. This creates a user-friendly experience.
- Ensuring cross-browser compatibility: When developing with JavaScript, make sure your code works consistently across different browsers (Chrome, Firefox, Safari, etc.). Modern JavaScript frameworks like React or Vue.js can help with this, but you’ll need to ensure the necessary libraries are correctly integrated. Testing on different browsers is crucial for a consistent user experience.
// Example JavaScript code (for a basic button):
window.onscroll = function()
if (document.body.scrollTop > 20 || document.documentElement.scrollTop > 20)
document.getElementById("backToTopBtn").style.display = "block";
else
document.getElementById("backToTopBtn").style.display = "none";
;
// ... (rest of the JavaScript code to handle button click and smooth scrolling)
This code snippet demonstrates how to display and hide the button based on the user’s scroll position. Remember to add the button element with the ID “backToTopBtn” in your HTML.
Server-Side Implementation with Custom Code
Server-side implementation is a more involved process, requiring modifications to your Blogger blog’s template. This method is generally more complex than the client-side approach, but it can be necessary for more advanced functionalities. The process involves modifying your Blogger theme’s template files.
- Modifying the theme’s HTML: This involves inserting the necessary HTML code to include the “Back to Top” button into your template. This can be done using custom CSS and JavaScript.
- Using server-side scripting languages: For more advanced implementations, server-side scripting languages like PHP, Python, or Ruby can be used. These can be helpful for handling dynamic content or database interactions.
- Integrating with your existing theme: Carefully integrate the new code into your existing theme to avoid breaking existing functionality. This is critical to prevent unexpected outcomes or errors on your blog.
Responsive Table for Back to Top Button Styles
Comparing different back-to-top button styles can help you make an informed decision. A well-designed table can illustrate these differences effectively. Here’s a suggested structure:
Style | Description | Pros | Cons |
---|---|---|---|
Simple Button | A basic button with minimal design. | Easy to implement, loads quickly. | Might look less appealing to users. |
Animated Button | A button with a smooth animation on hover or click. | Improved user experience, visually appealing. | Might slightly slow down page load times. |
Floating Button | A button that floats to the right of the screen as the user scrolls down. | Always visible, intuitive for users. | Might look intrusive if the design isn’t well-thought-out. |
This responsive table can be easily adapted to fit various screen sizes. Consider using CSS frameworks like Bootstrap or Tailwind CSS for added responsiveness.
Customizing the “Back to Top” Button’s Appearance and Functionality
Alright, fellow bloggers! Now that we’ve got the “Back to Top” button planted firmly on our pages, let’s spice things up a bit. We’re not just talking about a basic, unassuming button here; we’re talking about a button that screams “user-friendly” and “stylish.” Think of it as the finishing touch to a perfectly crafted blog post.This section dives deep into the art of customizing your button’s look and feel, and adding some cool animations to make it truly stand out.
We’ll explore different ways to make it pop, from vibrant colors to smooth scrolling effects.
Customizing the Button’s Visual Appearance
To make your “Back to Top” button a true reflection of your blog’s aesthetic, you need to tailor its appearance. This is where CSS styling comes into play. You can adjust colors, sizes, and even incorporate icons to create a visually appealing element.
.back-to-top
position: fixed;
bottom: 20px;
right: 20px;
background-color: #3498db; /* Example color
-/
color: white;
border: none;
padding: 10px 15px;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
opacity: 0.8;
transition: opacity 0.3s;
.back-to-top:hover
opacity: 1;
.back-to-top:focus
Artikel: none;
This CSS code snippet positions the button at the bottom right of the page, with a blue background, white text, and rounded corners. Hovering over it increases the opacity, making it more noticeable. Adjust the values for `bottom`, `right`, `background-color`, `font-size`, etc., to perfectly match your blog’s theme.
Adding Smooth Scrolling Animation
Let’s elevate the button’s functionality by incorporating smooth scrolling. This provides a more engaging user experience, making the “Back to Top” button feel more responsive.
document.querySelector('.back-to-top').addEventListener('click', function(event)
event.preventDefault();
window.scrollTo(
top: 0,
behavior: "smooth"
);
);
This JavaScript code snippet ensures that clicking the button smoothly scrolls the user back to the top of the page. The `behavior: “smooth”` is the key to creating that silky-smooth scrolling experience.
Adjusting Button Visibility
The button’s visibility should be dynamic, appearing only when needed. This prevents it from cluttering the screen unnecessarily.
window.addEventListener('scroll', function()
if (window.pageYOffset > 100)
document.querySelector('.back-to-top').style.display = 'block';
else
document.querySelector('.back-to-top').style.display = 'none';
);
This JavaScript code makes the button appear when the user scrolls down 100 pixels. Adjust the threshold (100 pixels in this example) to fine-tune when the button becomes visible.
Animation Effects
Here’s a table showcasing different animation effects you can implement.
Animation Effect | Description | Example (CSS) |
---|---|---|
Fade-in/Fade-out | Button fades in on scroll and fades out when near the top. |
`.back-to-top opacity: 0; /* …other styles… -/ window.addEventListener(‘scroll’, function() // … visibility check … document.querySelector(‘.back-to-top’).style.opacity = window.pageYOffset / 500; );` |
Slide-in/Slide-out | Button slides in from the right and slides out when near the top. |
`.back-to-top transform: translateX(50px); /* …other styles… -/ window.addEventListener(‘scroll’, function() // … visibility check … document.querySelector(‘.back-to-top’).style.transform = `translateX($-(window.pageYOffset / 10)px)`; );` |
These are just a few examples; the possibilities are virtually endless. Remember to tailor these effects to your blog’s unique style.
Troubleshooting Common Issues and Best Practices
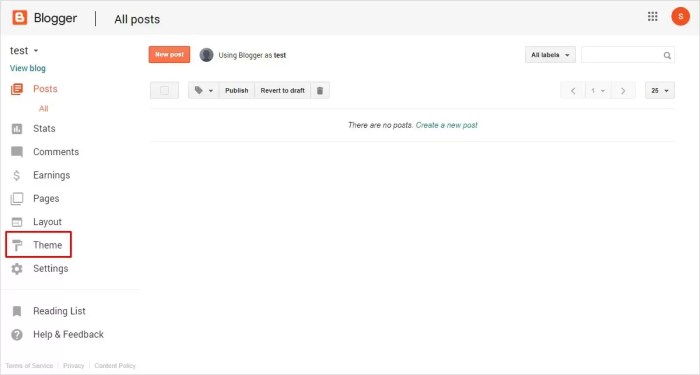
Adding a “Back to Top” button to your Blogger blog can be a lifesaver for readers, making your site more user-friendly. However, sometimes things don’t go as smoothly as planned. This section dives into common problems, providing solutions and best practices to ensure your button works flawlessly and enhances the user experience.
Implementing a smooth, accessible, and responsive “Back to Top” button requires careful consideration. It’s not just about adding a button; it’s about integrating it seamlessly with your blog’s design and user flow, making it an intuitive part of the navigation.
Theme and Plugin Compatibility Issues
Integrating the button might clash with your theme or plugins, leading to unexpected behaviors or visual glitches. One common issue is the button overlapping content or not appearing correctly in specific areas of the page. This often arises from conflicts between the button’s CSS and the theme’s styling. To troubleshoot, carefully examine the theme’s CSS file. Identify potential conflicts and modify the button’s CSS to resolve them, making sure to avoid overriding essential theme styles.
If the problem persists, consider using a custom CSS file for the button, keeping the theme’s CSS intact. This is a better practice, allowing easier future maintenance and minimizing unintended side effects on your theme.
Responsiveness and Accessibility
A great “Back to Top” button must work seamlessly across various screen sizes. Poor responsiveness can result in the button being hidden, overlapping other elements, or appearing in an awkward position. Testing on different devices and screen resolutions is crucial to catch such issues early. Accessibility is equally important; ensure the button is visible and operable for users with disabilities, including those using screen readers.
Use clear and concise text labels.
Responsiveness Testing Considerations
Screen Size | Browser | Device | Expected Behavior |
---|---|---|---|
Mobile (e.g., iPhone 12, Samsung Galaxy S22) | Chrome, Safari, Firefox | Smartphone | Button should be easily visible and clickable, not obstructed by other elements. Should be readily accessible from the bottom of the page. |
Tablet (e.g., iPad Pro, Samsung Galaxy Tab S8) | Chrome, Safari, Firefox | Tablet | The button should remain visible and functional, and not overlap with content. The size and placement should be appropriate for the larger screen. |
Desktop (e.g., 1920×1080, 2560×1440) | Chrome, Firefox, Edge | Laptop, Desktop | The button should be easily accessible from the bottom of the page and not overlap with content, or obstruct other important page elements. |
Large Desktop (e.g., Ultrawide monitor) | Chrome, Firefox, Edge | Laptop, Desktop with Ultrawide screen | The button should maintain appropriate size and placement, avoiding any overlapping issues. |
Accessibility Best Practices, How to add back to top to blogspot blog
For optimal accessibility, consider these points:
- Use descriptive anchor text for the button. Instead of “Top,” use “Back to Top.” This improves understanding for users relying on screen readers.
- Ensure sufficient contrast between the button’s text and background. This helps users with visual impairments discern the button from surrounding elements.
- Implement proper ARIA attributes (if necessary) to clearly communicate the button’s function to assistive technologies.
- Avoid using complex or unusual animations for the button, as these can be distracting or confusing for some users.
Epilogue
Adding a “Back to Top” button to your Blogger blog is now a breeze! By following the methods and best practices Artikeld in this guide, you’ll significantly enhance the user experience on your blog. Remember to prioritize responsiveness, accessibility, and troubleshooting for a smooth and engaging visitor journey.
Clarifying Questions
How do I choose the right method for adding the button?
The best method depends on your technical skills and desired level of customization. For simple implementations, JavaScript is often sufficient. If you’re comfortable with coding, custom solutions offer more flexibility.
What if my theme conflicts with the button?
Sometimes, themes can interfere with custom elements. Carefully review the theme’s documentation and ensure compatibility. If the problem persists, consider adjusting the button’s CSS or using a more compatible theme.
How can I make the button look better?
Use CSS styling to customize the button’s appearance, including color, size, and icon. You can also add animations for a more engaging user experience.
Can I make the button appear only when needed?
Yes, you can use JavaScript to set conditions for the button’s visibility. It will appear only when the user scrolls a certain distance down the page.