Class validator how to override rule? Level up your coding game with this deep dive. We’re breaking down the nitty-gritty of customizing validation rules in your class validators, from simple tweaks to complex overrides. Get ready to master the art of crafting flexible and powerful validation systems.
This guide meticulously details the process of overriding validation rules within a class validator, equipping you with the knowledge and tools to handle various scenarios with ease. We’ll cover everything from the foundational concepts to advanced techniques, making complex procedures simple and straightforward.
Introduction to Class Validators
Class validators are a crucial component in object-oriented programming, providing a structured and organized way to ensure data integrity within a class. They act as gatekeepers, validating the data being assigned to object attributes before it’s stored or used. This prevents inconsistencies and errors in your application, leading to more robust and reliable software.Class validators offer a streamlined approach to input validation, simplifying the process of checking data types, ranges, and other constraints.
This validation occurs during object creation or attribute modification, allowing for immediate feedback and preventing invalid data from propagating through the system.
Common Use Cases
Class validators are widely used across various programming scenarios. They are particularly valuable in applications dealing with user input, data processing, and business logic. Validating user inputs like email addresses, phone numbers, or dates helps prevent unexpected behavior and security vulnerabilities. They also play a vital role in ensuring data consistency within data models, preventing database corruption and ensuring accurate data processing.
- Data Input Validation: Ensuring user-provided data adheres to predefined rules (e.g., email format, password complexity). This prevents incorrect data from being stored or processed, leading to a more reliable application.
- Data Type Enforcement: Guaranteeing that attributes of a class hold data of the correct type. For example, ensuring an age attribute is an integer and not a string, preventing unexpected errors during calculations or comparisons.
- Range and Format Validation: Restricting attribute values to specific ranges or formats. For example, validating a price value to be within a specific range or validating a date format to conform to a particular standard. This ensures data accuracy and consistency.
Benefits of Using Class Validators
Implementing class validators provides several significant advantages. They enhance the overall reliability and maintainability of your code. Validation rules are encapsulated within the class, making the code more organized and readable. This modular approach also simplifies testing and debugging, as validation logic is contained in a single location.
- Improved Data Integrity: Preventing invalid data from entering the system, leading to fewer errors and more predictable application behavior.
- Enhanced Code Maintainability: Centralizing validation rules within the class structure, making it easier to update or modify validation logic without affecting other parts of the codebase.
- Reduced Debugging Time: Identifying data issues early in the development process, enabling faster identification and resolution of problems.
- Increased Application Robustness: Handling unexpected inputs gracefully and preventing critical errors from occurring, leading to a more stable application.
Example Implementation
This example demonstrates a simple class validator for validating user input.“`javapublic class User private String name; private int age; public User(String name, int age) this.name = name; this.age = age; validate(); private void validate() if (name == null || name.isEmpty()) throw new IllegalArgumentException(“Name cannot be null or empty.”); if (age <= 0) throw new IllegalArgumentException("Age must be a positive integer."); // Getters for name and age (omitted for brevity) ``` This `User` class includes a constructor that validates the `name` and `age` attributes. If either validation fails, an `IllegalArgumentException` is thrown. This ensures that only valid user objects are created. Note that error handling is a critical component of robust validation.
Understanding Validation Rules
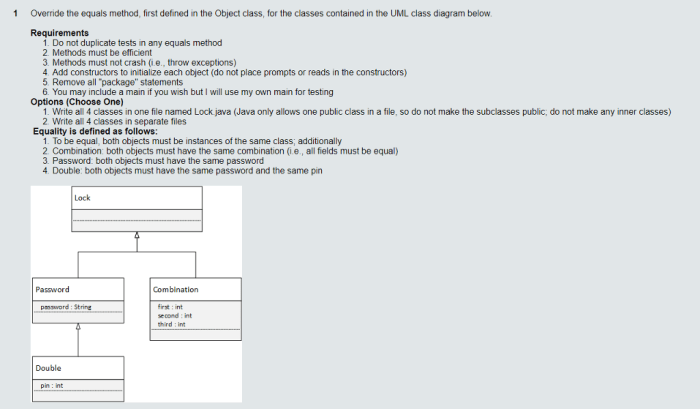
Validation rules are the cornerstone of robust class validators.
They define the criteria that data must meet to be considered valid. Correctly implemented validation rules prevent invalid data from entering your system, ensuring data integrity and application stability. This section delves into the structure, types, and practical implementation of validation rules within a class validator.Validation rules are meticulously crafted to specify precisely what constitutes valid input. These rules are not arbitrary; they are based on the expected format, range, and constraints of the data being validated.
This meticulous approach ensures that only appropriate data is accepted, mitigating the risk of errors and unexpected behavior in your application.
Validation Rule Structure
Validation rules typically consist of a field name and a validation method. The field name identifies the data being validated, while the validation method dictates the criteria for validity. For instance, a rule might specify that the “email” field must be a valid email address according to a specific format. This structured approach enables a clear and unambiguous definition of validation criteria.
Validation Rule Types
A variety of validation rules can be employed within a class validator, each catering to specific data requirements. Understanding the nuances of these types allows for a tailored approach to data validation.
- Required Rules: These rules ensure that a particular field is not left blank. This is crucial for fields that are essential to the data’s completeness, like names or addresses.
- Length Rules: These rules specify the acceptable length of a string. This might involve a minimum or maximum length, or both. Length rules are critical for controlling the size of data elements like passwords or usernames.
- Format Rules: These rules dictate the expected structure of data. Examples include validating email addresses, phone numbers, or credit card numbers.
- Range Rules: These rules specify a valid numerical range for a field. This is often used for quantities, prices, or other numerical data. They ensure data falls within an acceptable threshold.
- Custom Rules: These rules allow for the implementation of complex validation logic not covered by predefined rules. This is especially useful for scenarios with unique data constraints.
Examples of Validation Rule Implementation, Class validator how to override rule
Let’s illustrate the implementation of different validation rules.
- Required Field: A validation rule for a “name” field might require it to not be empty.
- Length Rule: A “password” field might require a minimum length of 8 characters.
- Format Rule: A “phone number” field could be validated to match a specific phone number pattern.
- Range Rule: An “age” field could be validated to ensure it’s within a specific age range.
Comparison of Validation Rule Types
The table below summarizes different validation rule types and their corresponding use cases.
Rule Type | Description | Use Case | Example |
---|---|---|---|
Required | Ensures a field is not empty | Essential fields like names, addresses | Name field cannot be blank |
Length | Specifies minimum/maximum length | Passwords, usernames, descriptions | Password must be 8-20 characters long |
Format | Validates data structure | Emails, phone numbers, dates | Email must follow valid format |
Range | Validates numerical range | Prices, quantities, ages | Age must be between 0 and 120 |
Overriding Validation Rules
Class validators provide a structured way to enforce data integrity. However, sometimes predefined validation rules might not perfectly suit specific use cases. This necessitates the ability to override these rules to tailor the validation process to unique requirements.Overriding validation rules allows developers to customize the validation logic for specific scenarios. This is particularly useful when standard validation doesn’t account for exceptional circumstances or business-specific requirements.
Reasons for Overriding Validation Rules
Custom validation is often necessary when standard validation rules are insufficient for a particular data input. For instance, a user might need to enter a specific date format that differs from the default date format supported by the validation library. Another example might be a field that accepts a range of values beyond the predefined acceptable values.
Situations Where Overriding is Useful
Overriding is essential in situations where standard validation rules do not align with application-specific requirements. This can be crucial for handling unique input formats, specialized data types, or implementing custom business logic during validation.
Example Scenarios
- Date Format Customization: A system might require a specific date format (e.g., YYYY-MM-DD) that deviates from the default format supported by the validator. Overriding allows the validation rule to accommodate this unique requirement.
- Range Validation Extension: A field might need to accept a range of values beyond the default range defined by the validation rule. Overriding the validation rule allows for flexible value acceptance within a custom range.
- Custom Data Type Validation: The validator may not recognize a specific data type. Overriding the validation rule allows for the addition of custom validation logic for this data type.
Step-by-Step Guide to Overriding a Validation Rule
This guide Artikels the general steps for overriding a validation rule within a class validator. Specific implementations may vary based on the chosen framework or library.
- Identify the Rule to Override: Determine which validation rule needs modification. Carefully review the existing validation rules to pinpoint the rule that needs customization.
- Locate the Rule Definition: Find the code section where the validation rule is defined within the class validator. This usually involves examining the class’s source code or documentation.
- Create a Custom Validation Method: Develop a new method that encapsulates the customized validation logic. This method will replace the original validation logic for the specific case.
- Replace the Original Validation Logic: Modify the existing validation rule to call the custom validation method instead of the original validation logic.
- Test the Custom Validation: Thoroughly test the overridden validation rule to ensure that it correctly handles the desired scenarios.
Illustrative Example (Conceptual)
Imagine a class validator that checks for email addresses. The standard rule might validate based on a simple pattern. To handle a specific company email format, the developer would create a custom validation method that validates against a more complex pattern specific to that format.
Implementing Rule Overrides
Implementing overrides for validation rules allows you to tailor the validation process to specific needs. This is crucial for handling complex data structures and ensuring data integrity while maintaining flexibility. Overrides are essential for adapting to unique situations, where standard validation rules might not suffice.Implementing validation rule overrides can involve modifying the validation logic to accommodate exceptions or specific cases.
This process ensures that data meets the criteria set for each particular situation.
Required Rule Overrides
Customizing required field validation often involves specifying exceptions to the default rule. For example, a field might be optional under certain conditions, such as when another field is populated with a specific value.
- To override the required rule, you might check the value of another field. If the other field meets a specific criterion, the required field can be exempted from validation.
- The implementation typically involves conditional logic within the validation function, ensuring the required field is only validated when necessary.
Length Rule Overrides
Length rules can be overridden to accommodate varying input lengths based on specific conditions. For instance, a username might have a different maximum length than a comment.
- The override implementation typically involves checking for specific criteria to determine the appropriate length limit for a given input.
- Conditional logic within the validation function helps to set the maximum length according to the criteria.
Format Rule Overrides
Format rules can be overridden for various data types, such as dates, email addresses, or phone numbers. For instance, a date format might need to accommodate different regional conventions.
- The override typically involves creating a custom validation function to handle the specific format requirements.
- This function might employ regular expressions or other validation techniques to check the input against the specific format.
Custom Rule Overrides
Custom validation rules can be overridden to accommodate unique validation requirements that aren’t covered by standard rules. Consider a rule for validating the format of a complex data structure.
- Overriding custom rules involves creating a new validation function that replaces or extends the existing one.
- This involves writing the custom logic within the validation function to enforce the required format for the specific data type.
Table of Validation Rule Override Steps
Validation Rule | Override Approach | Implementation Example (Conceptual) | Notes |
---|---|---|---|
Required | Conditional logic based on other fields | If field ‘otherField’ has value ‘X’, then ‘requiredField’ is not required. | Use conditional statements to check for exceptions. |
Length | Conditional length limit based on context | If field ‘type’ is ‘short’, then max length is 10; otherwise, max length is 20. | Adjust maximum length based on conditions. |
Format | Custom validation function with specific format check | Validate date format using a custom function (e.g., `isValidDate(dateString, format)`) | Create a function for the particular format. |
Custom | Create a new function to replace/extend the existing custom validation | Implement a function to check for the specific format of a complex data structure | Replace the old function with the new custom validation. |
Handling Errors and Exceptions
Proper error handling is crucial for robust class validators. Incorrectly handled exceptions can lead to application crashes or unexpected behavior, impacting user experience and data integrity. Thorough error management ensures smooth operation and reliable data validation.Effective error handling in validation rules is vital for maintaining application stability. Catching and reporting errors appropriately prevents unexpected crashes, facilitates debugging, and improves the user experience by providing clear and informative feedback.
By following best practices for exception handling, developers can ensure their validation logic is resilient to various scenarios.
Handling Validation Errors During Rule Overrides
When overriding validation rules, carefully consider how to manage errors arising from the overridden logic. A custom override might introduce conditions that weren’t anticipated in the original validation rule, potentially leading to errors. Careful error handling within the overridden rule is critical to preventing unexpected application behavior. It’s essential to anticipate potential issues and craft error messages that clearly explain the nature of the problem to the user or the application.
This allows for quick diagnosis and resolution.
Best Practices for Reporting Errors
Clear and concise error messages are essential for effective debugging and user feedback. When a validation error occurs, providing specific details about the violated rule and the offending data helps users understand the problem quickly. This detailed information assists in troubleshooting and fixing the issue, minimizing the time spent on resolving errors. For instance, if a rule requires a positive integer, an error message like “Field ‘age’ must be a positive integer” is much more helpful than a generic “Validation error.” Specific messages are essential for maintainability and problem-solving.
Gracefully Handling Exceptions Related to Rule Overrides
Exception handling during rule overrides is crucial for maintaining application stability. Exceptions can arise from various sources, such as invalid input data, resource limitations, or unexpected conditions. To prevent application crashes, implement robust exception handling mechanisms. This ensures that the application continues to operate even when an unexpected problem occurs. Appropriate exception handling involves catching potential exceptions, logging them for debugging purposes, and providing a graceful response to the user or the application.
Best Practices for Exception Handling During Validation Rule Overrides
Effective exception handling during validation rule overrides requires a proactive approach. Implement try-catch blocks to capture exceptions. Log exceptions thoroughly to facilitate debugging. Provide informative error messages to the user, while also taking necessary actions to prevent data loss or further issues. Finally, consider the context of the exception and implement specific handling for different types of exceptions.
Advanced Techniques and Considerations
Overriding validation rules in class validators requires careful consideration of various techniques and potential pitfalls. Choosing the right approach depends on the complexity of the validation logic and the desired maintainability of the code. This section delves into advanced strategies, potential limitations, and factors to consider when implementing rule overrides.
Inheritance for Rule Overrides
Inheritance provides a straightforward way to extend existing validation rules. By creating a subclass of the validator class, developers can modify or add validation logic to the existing rules. This approach is particularly useful for scenarios where a validation rule needs a small modification.
Composition for Rule Overrides
Composition is another powerful technique for overriding validation rules. Instead of inheriting from the existing validator class, a new class can encapsulate the overridden logic. This approach is beneficial when multiple validation rules need customization, or when the existing validator class is not intended to be extended. It promotes code modularity and reduces the risk of unintended side effects when extending the validator class.
Comparing Inheritance and Composition
Feature | Inheritance | Composition |
---|---|---|
Code Structure | Extends the existing class | Creates a new class, encapsulating logic |
Flexibility | Limited, focused on extending existing functionality | High, allows for more complex and independent logic |
Maintainability | Can become complex with many overrides | Generally more maintainable, modular code |
Extensibility | Can be cumbersome with many levels of inheritance | Easy to extend, new components can be added without affecting existing parts |
Pitfalls and Limitations of Rule Overrides
Overriding validation rules can introduce several potential pitfalls. Incorrectly implemented overrides can lead to unexpected behavior or vulnerabilities. Careful consideration of the existing validation logic and potential interactions with other parts of the system is crucial. Thorough testing and documentation are essential for mitigating these risks.
Factors to Consider When Choosing an Override Strategy
Several factors influence the optimal strategy for overriding validation rules. These factors include the number of rules needing modification, the complexity of the overridden logic, and the overall architecture of the application. Maintaining a clear separation of concerns, using appropriate design patterns, and writing thorough unit tests are critical to avoid unintended consequences. Consider the potential impact of changes on other parts of the system when making decisions about overriding validation rules.
Example of Inheritance
A simple example of overriding a rule using inheritance might involve a `UserValidator` class. A subclass, `AdminUserValidator`, could inherit from `UserValidator` and override the `validateEmail` method to add specific constraints for admin users.
Example of Composition
In composition, a `UserValidator` class might use a `CustomEmailValidator` to handle email validation. This allows independent modifications of email validation logic without altering the core `UserValidator` class.
Example Scenarios: Class Validator How To Override Rule
Overriding validation rules in class validators is crucial for adapting validation logic to specific use cases. This section provides practical examples showcasing how to customize validation rules for various scenarios, including specific data types and error handling.Understanding how to override validation rules is vital for flexibility and maintainability. By customizing the validation process, developers can adapt the application to unique requirements without modifying the core validation logic.
Real-World Application Scenario
Consider an e-commerce application where product prices must be positive and not exceed a certain limit. A default validation rule might enforce this. However, during promotional sales, a specific product category might require an exception to the price limit.
- The standard validation rule might prevent the price from being negative or above 1000 USD. However, for a promotional product (e.g., “Summer Sale” category), this price limit should be 2000 USD.
- A class validator can be overridden to check the product category. If the product belongs to “Summer Sale,” the price limit is 2000 USD, otherwise, the standard 1000 USD limit applies.
Data Type-Specific Override
A validation rule might typically validate email addresses. However, an application might need to handle different data types (e.g., phone numbers) within the same class.
- For phone numbers, a different format is required than for email addresses. For instance, the validation for phone numbers could involve checking for a specific format (e.g., 10-digit format).
- A custom validation rule can be implemented that is invoked when the data type is identified as a phone number, applying specific validation logic. The validation framework should allow the user to identify the data type for the override to work.
Error Handling During Overrides
Handling errors during overrides is critical for robustness. Consider a scenario where the overridden validation rule encounters an unexpected exception.
- The validation framework should be designed to gracefully handle exceptions raised by the overridden rule. The error should be reported to the user or logged for debugging, preventing the application from crashing.
- The framework should provide a mechanism for handling the exceptions thrown during the override process, ensuring the application’s stability and reliability.
Specific Validation Framework Example (Hypothetical)
Let’s imagine a hypothetical validation framework called “ValiFrame.” ValiFrame allows for overriding validation rules using custom methods.
Validation Rule | Default Implementation | Overridden Implementation | Error Handling |
---|---|---|---|
Price Validation | Price > 0 and Price < 1000 | If ProductCategory = “Summer Sale”, Price < 2000, otherwise Price < 1000 | Throws ValidationException if price is invalid. Catches the exception and logs the error. |
Email Validation | Valid email format | If DataType = “PhoneNumber”, validates phone number format, otherwise validates email format. | Throws InvalidFormatException if format is incorrect. Catches the exception and provides a user-friendly error message. |
Date Validation | Valid date format | If DataType = “DateOfBirth”, checks for valid age, otherwise checks date format. | Throws InvalidDateException if the date is invalid. Catches the exception and returns an appropriate error message. |
Best Practices and Recommendations
Implementing validation rule overrides effectively requires careful consideration to ensure maintainability, reusability, and code clarity. Improper implementation can lead to complex, hard-to-understand code and introduce vulnerabilities. This section details best practices to avoid these pitfalls.Effective override strategies are crucial for maintaining flexibility and extensibility in validation logic without compromising the robustness of the system. Adhering to these best practices will lead to more maintainable and scalable applications.
Implementing Overrides with Clarity
Clear naming conventions and well-documented code are essential for maintaining readability. Choose descriptive names for override methods and classes, and provide comprehensive comments explaining the rationale behind each override. This makes it easier for other developers (and yourself in the future) to understand and maintain the code. Using a consistent coding style, like following established naming conventions or using linters, will also contribute to overall code quality.
Maintaining Maintainability During Overrides
Validation rules should be designed with maintainability in mind. Avoid tightly coupled code where modifications in one part of the system significantly impact another. Favor loose coupling through interfaces and abstract classes, allowing for easier modifications without affecting other parts of the application. Implementing modular validation logic, separating validation rules into smaller, self-contained modules, will significantly improve maintainability and scalability.
Creating Reusable Validation Logic
Developing reusable validation components will enhance code maintainability and prevent redundant code. Abstraction is key. Define abstract validation classes or interfaces that encapsulate common validation logic. Subclasses can then override specific rules without repeating the base implementation. This approach encourages code reuse and makes modifications easier.
Important Considerations for Overrides
Effective validation override implementation necessitates careful consideration of several factors:
- Error Handling: Implement comprehensive error handling mechanisms within override methods. This will help identify and manage issues that arise during the validation process. This includes logging exceptions and providing meaningful error messages to the user.
- Performance: Overrides should not significantly impact the performance of the validation process. Avoid computationally expensive operations within the override methods. Consider caching frequently used data to optimize performance.
- Security: Carefully review the security implications of any changes to validation rules. Ensure that overrides do not introduce vulnerabilities that could compromise the system. Sanitize user input and validate data against known threats.
- Testing: Thoroughly test all override implementations. Develop comprehensive test cases that cover various scenarios, including boundary conditions, edge cases, and unexpected input. Unit testing is crucial to ensure that overrides function as expected and do not introduce unintended side effects.
Ultimate Conclusion
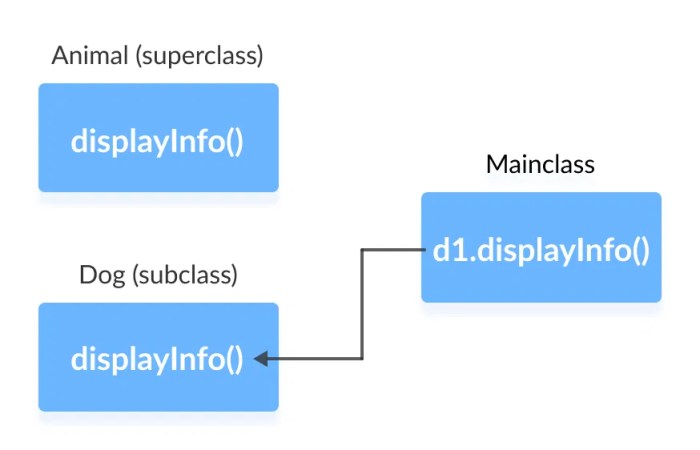
So, you’ve conquered the art of overriding validation rules within your class validator! You’ve learned how to customize validation, handle errors gracefully, and make your code both flexible and maintainable. This knowledge is your superpower for building robust applications. Now go forth and validate with confidence!
FAQ Explained
What are some common reasons to override validation rules?
Sometimes, built-in validation rules aren’t quite right for your specific needs. You might need to adjust length requirements, alter formatting standards, or add completely new rules based on unique application logic. This is where overriding comes in.
How do I handle errors during validation rule overrides?
Implementing robust error handling is crucial. Always provide informative error messages and ensure your code gracefully handles exceptions that might arise during overrides. This prevents your application from crashing and provides users with helpful feedback.
Can I reuse override logic for different validation rules?
Absolutely! Designing reusable functions or classes for your validation overrides can significantly improve code organization and maintainability. You can even create a library of reusable validation logic for future projects.
What are some best practices for maintaining clarity when overriding rules?
Document your overrides thoroughly. Use descriptive variable names and comments to explain the logic behind your custom rules. Following a consistent naming convention will make your code easier to read and understand for yourself and others in the future.