How to assign event handler in c builder – How to assign event handler in C# Builder? This guide dives deep into the world of event handling, a crucial aspect of building interactive C# Builder applications. We’ll explore fundamental concepts, different event types, and practical implementation strategies, ensuring you master event handling for your projects. From simple buttons to complex custom events, this guide covers it all.
This detailed walkthrough will equip you with the knowledge to create robust and responsive C# Builder applications. We’ll cover everything from basic event handling to advanced techniques like multi-threading and error handling. Mastering these techniques will significantly enhance your application development skills.
Introduction to Event Handling in C# Builder
Salam! Event handling is a crucial aspect of building interactive C# Builder applications. It allows your application to respond dynamically to user actions or internal system changes. Imagine a button click; event handling dictates how the application reacts to that click. Understanding events, event handlers, and delegates is key to creating responsive and user-friendly applications.
Fundamentals of Event Handling
Event handling in C# Builder is centered around the concept of events, which signal the occurrence of an action. Event handlers are the code blocks that respond to these events. Delegates act as the intermediaries between events and handlers, enabling the connection and execution of the handler code. This structured approach enhances code maintainability and allows for modular design, a hallmark of good software engineering.
Events
Events are signals that indicate an action has taken place within the application, like a button click or a form load. They are a mechanism to decouple different parts of the application, allowing them to interact without direct knowledge of each other’s internal workings. This decoupling is vital for creating more flexible and maintainable code.
Event Handlers
Event handlers are the specific code blocks that respond to a particular event. They contain the instructions that the application should execute when the event occurs. Handlers are crucial for defining the application’s response to user actions and internal events. By placing handlers, the application can be responsive to user interaction and internal changes.
Delegates
Delegates are like pointers to methods. They act as a bridge between events and handlers, enabling events to call the appropriate handler code. This connection is crucial for enabling the dynamic response to events, allowing the application to be interactive. Think of delegates as the messengers carrying the event information to the correct handler.
Example: A Simple Button Click, How to assign event handler in c builder
Let’s illustrate a simple button click event. The following example demonstrates how to create an event handler for a button click in a C# Builder application:“`C#// Event handler for the button clickprivate void button1Click(object sender, EventArgs e) MessageBox.Show(“Button Clicked!”);“`This code defines a method `button1Click` that will execute when the button is clicked. The `sender` object refers to the control that generated the event, and `EventArgs` contains additional information about the event.
Typical Event-Handling Setup
A typical event-handling setup in C# Builder involves these steps:
- Declare the Event: Define the event in the component class where the event originates.
- Create the Event Handler: Define the method that will handle the event. This method is the code that will execute in response to the event.
- Connect the Handler: Use the appropriate syntax to associate the event handler with the event. This step connects the event and the handler so that when the event occurs, the handler is executed.
This structured approach ensures a clear and manageable flow of execution in response to user actions or application changes. This structured approach promotes better code organization and readability.
Different Types of Events in C# Builder
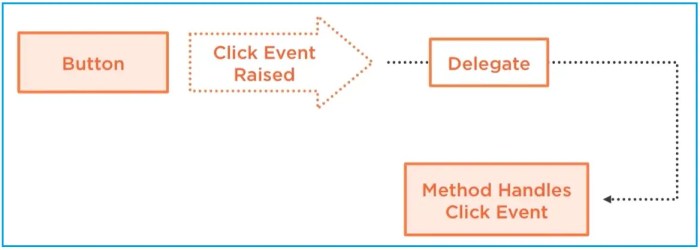
Salam kenal, and welcome to this exploration of event handling in C# Builder. Understanding the various types of events is crucial for crafting responsive and interactive applications. This section will delve into system-defined and custom events, illuminating their differences and providing practical examples of how to handle them effectively.Event handling in C# Builder, like other programming paradigms, is a fundamental concept for building interactive applications.
It allows you to react to specific actions or occurrences within your program, enabling dynamic behavior and a more user-friendly experience.
Common Types of Events
Familiarizing yourself with the common types of events is vital for writing effective event handlers. These pre-defined events, part of the C# Builder framework, facilitate responsiveness to standard user actions and system changes.
Handling Custom Events
Developing your own custom events empowers you to create specific reactions to unique circumstances within your application. This customization allows tailored responses to situations not covered by standard events. By defining your own events, you enhance the modularity and maintainability of your code.
System-Defined vs. User-Defined Events
System-defined events are inherent parts of the C# Builder framework, automatically triggering in response to standard user interactions or system changes. These are pre-built, so you don’t need to define them explicitly. In contrast, user-defined events are events you create specifically to respond to unique actions or occurrences in your application. This customization allows for tailored reactions to events not handled by built-in events.
Event Arguments and Usage
Event arguments provide the context for an event. They carry essential data about the event, such as the source of the event, the event’s specifics, or the object affected. Using event arguments correctly allows you to react to the event’s circumstances with precision and appropriateness. They are vital for tailoring your response to the precise event details.
Table of Event Types and Arguments
The table below showcases common event types and their associated arguments. This provides a clear reference for understanding the structure of event handling in C# Builder.
Event Type | Event Source | Event Argument Type | Description |
---|---|---|---|
Form Show | TForm | TShowMessageEventArgs | Triggered when a form is displayed. |
Button Click | TButton | TMouseEvent | Triggered when a button is clicked. |
Timer Tick | Ttimer | TEvent | Triggered at specified intervals. |
Custom Event: DataUpdated | CustomComponent | TDataUpdateEventArgs | Triggered when data is updated in a custom component. |
Implementing Event Handlers
Salam kenal, fellow developers! Now that we’ve explored the world of events and their various types in C# Builder, let’s dive into the practical side of things: implementing event handlers. This crucial step allows our applications to respond dynamically to user interactions and internal system changes.
Attaching Event Handlers
To hook up an event handler, we essentially tell the component that triggers the event (like a button click) to execute a specific piece of code when the event occurs. This is done by associating a method with the event. This method, often called an event handler, contains the instructions for how our application will react. Understanding how to connect these pieces is key to building responsive and interactive applications.
Defining and Implementing Event Handlers
There are several ways to define and implement event handlers in C# Builder, each with its own nuances and benefits. Here are a few common approaches:
- Using Method Names: This is the most straightforward approach. We simply specify the name of an existing method that should be executed when the event occurs. This approach works well for handlers that have a clear, concise purpose.
- Using Lambda Expressions: Lambda expressions offer a more concise way to define short, anonymous methods directly within the event handler assignment. This is particularly useful when the handler logic is simple and doesn’t warrant a separate named method. This approach is a powerful tool for rapid prototyping and concise code.
- Using Anonymous Methods: Similar to lambda expressions, anonymous methods allow for defining event handlers without explicitly naming the method. They provide more flexibility than lambda expressions when the handler logic is more complex.
Examples for User Interface Controls
Let’s illustrate with examples for common user interface controls:
- Button Click Event: Imagine a button named ‘btnClickMe’. To execute a method called ‘HandleButtonClick’ when the button is clicked, we would use code like this:
btnClickMe.Click += HandleButtonClick;
This line attaches the HandleButtonClick method to the Click event of the button. This approach is quite straightforward and efficient for most button-related events. - Text Box Text Changed Event: When a user types in a text box, you can respond to the change in the text. The code for this looks similar to button event handling:
txtMyTextBox.TextChanged += (sender, e) => // Handle the text change ;
This code uses a lambda expression to handle the text change event, updating the application’s state in real time.
Implementing Event Handlers for Custom Events
Custom events are a powerful tool for creating modular applications. To implement event handlers for custom events, we need to define the event in the class where it originates, then use the `+=` operator to attach the handler.
- Defining a Custom Event: The first step is to define a custom event in the class. This involves declaring an event of a specific type (often a delegate). This is the key to managing events within our application’s components.
- Creating Event Handlers: Next, we create methods that act as event handlers for our custom events. These handlers are often tied to specific actions within the class.
- Attaching the Handler: Finally, we use the `+=` operator to connect the event handler to the custom event. This establishes the link between the event trigger and the handler’s response.
Comparison of Handler Implementation Methods
The table below compares the different approaches for implementing event handlers.
Method | Implementation | Conciseness | Complexity |
---|---|---|---|
Method Names | Using named methods | Moderate | Moderate |
Lambda Expressions | Inline method definitions | High | Low |
Anonymous Methods | Anonymous method definitions | Moderate | Moderate |
Event Handling with Delegates
Event handling in C# Builder, like many other programming paradigms, leverages the power of delegates to create more flexible and maintainable event-driven architectures. Delegates act as pointers to methods, allowing you to pass methods as arguments to other methods. This capability is crucial in event handling because it enables you to dynamically attach and detach event handlers without modifying the event source code.
This approach promotes code reusability and modularity.
Role of Delegates in Event Handling
Delegates are fundamental to event handling in C# Builder because they act as a bridge between event-raising objects and event-handling methods. A delegate essentially defines a contract for a method; it specifies the method’s signature (return type and parameters). By using delegates, you can create event handlers that can be easily attached to and detached from events. This decoupling promotes loose coupling between components in your application.
Creating and Using Delegates
To create a delegate, you define a delegate type that specifies the method signature it can hold. The delegate type acts as a blueprint for methods that can be assigned to it. Once the delegate type is defined, you can create delegate instances by assigning a method to it.
Advantages of Using Delegates
Using delegates for event handling offers several key advantages:
- Flexibility: You can easily attach and detach event handlers without modifying the event source code. This promotes loose coupling, making your application more adaptable to changes.
- Maintainability: Code becomes more organized and easier to maintain as you can separate event handling logic from the event source.
- Extensibility: New event handlers can be added or removed without altering the event source. This flexibility enhances the application’s adaptability to future requirements.
- Reusability: Event handlers can be reused across multiple events or parts of the application.
Example: Implementing an Event Handler with a Delegate
This example demonstrates how to create a custom event and attach an event handler using a delegate.“`C#// Define a delegate typepublic delegate void MyEventHandler(object sender, EventArgs e);// Define a custom eventpublic class MyEventSource public event MyEventHandler MyEvent; public void RaiseMyEvent() if (MyEvent != null) MyEvent(this, EventArgs.Empty); // Example usagepublic class MyEventHandlerClass public void HandleMyEvent(object sender, EventArgs e) Console.WriteLine(“Event triggered!”); // Attaching the event handlerpublic class Example public static void Main(string[] args) MyEventSource source = new MyEventSource(); MyEventHandlerClass handler = new MyEventHandlerClass(); //Attach the event handler source.MyEvent += handler.HandleMyEvent; source.RaiseMyEvent(); “`
Attaching and Detaching Event Handlers using Delegates
The following code snippet demonstrates how to attach and detach event handlers using delegates:“`C#// Attaching the handlersource.MyEvent += handler.HandleMyEvent;//Detaching the handlersource.MyEvent -= handler.HandleMyEvent;“`These code snippets demonstrate the elegance and power of delegates in event handling. The ability to dynamically attach and detach handlers provides a powerful and flexible way to manage events in your applications.
Handling Multiple Events
Handling multiple events in C# Builder, like many other programming languages, allows a single control to respond to various user interactions or internal changes. This flexibility enhances application responsiveness and user experience. Understanding how to manage these events effectively is crucial for creating robust and interactive applications.
Strategies for Handling Multiple Events
Different strategies exist for managing multiple events triggered by a single control. The approach chosen depends on the specific requirements of the application and the nature of the events.
Handling Multiple Events with a Single Event Handler
Sometimes, multiple events share a common response. Using a single event handler for multiple events simplifies the code and reduces redundancy. This approach is particularly suitable when the actions triggered by different events are fundamentally similar.
- This method combines event handling logic for different events into a single function.
- The event handler needs to distinguish between the different event types within the handler itself, usually by checking the event object.
- This approach can lead to more concise code but might reduce code readability if the events have significantly different actions.
Handling Multiple Events with Separate Event Handlers
For events that require distinct actions, using separate event handlers for each event is often the preferred method. This approach improves code organization and clarity, making it easier to maintain and debug the application.
- Each event type has its dedicated event handler, improving code organization and readability.
- This method promotes better code maintainability as each handler is focused on a specific event type.
- The downside is that the code can become more verbose if there are many events to handle.
Best Practices for Effective Event Handling
Employing well-structured and organized event handling practices is crucial for maintaining code quality and ensuring smooth application functionality. Clear separation of concerns and appropriate naming conventions significantly contribute to better code maintainability.
- Use descriptive event names that clearly indicate the associated actions.
- Create distinct event handlers for each event type to maintain code clarity and maintainability.
- Consider the complexity of the actions associated with each event to determine the appropriate event handling strategy.
Event Handling Strategies Comparison
The following table Artikels different strategies for handling multiple events, highlighting their advantages and disadvantages:
Strategy | Description | Advantages | Disadvantages |
---|---|---|---|
Single Handler | One handler for multiple events. | Concise code; reduced code duplication | Potential for reduced code readability if events are dissimilar; complex logic within handler. |
Separate Handlers | Individual handlers for each event. | Improved code readability; better maintainability; easier debugging. | More verbose code; potential for code bloat with many events. |
Delegates | Using delegates to handle multiple events. | Provides flexibility in combining handlers. | Potentially complex for multiple events with varying actions. |
Event Aggregator Pattern | Centralized event handling. | Suitable for complex applications with numerous events. | Increased complexity for implementing the aggregator. |
Error Handling and Event Handling
Maintaining the stability and reliability of applications is crucial, especially when dealing with external events. Robust error handling within event handlers is essential to prevent unexpected crashes and ensure smooth application behavior. By anticipating and addressing potential errors, we can create more resilient and user-friendly software. This section dives into the practical aspects of integrating error handling into event handlers in C# Builder, focusing on strategies to mitigate potential issues and maintain application integrity.
Incorporating Error Handling within Event Handlers
Error handling within event handlers is a critical aspect of software development. Properly implemented error handling prevents program crashes, improves user experience, and aids in debugging. It’s essential to anticipate potential exceptions and provide mechanisms to gracefully manage them, minimizing the risk of application failures. This involves carefully evaluating the potential for errors during event processing and establishing appropriate responses to these errors.
Importance of Handling Exceptions within Event Handlers
Exceptions are inevitable during event processing, especially when interacting with external systems or user input. Ignoring these exceptions can lead to application crashes, data loss, or security vulnerabilities. By anticipating and handling potential exceptions within event handlers, we can ensure the application’s continued operation and maintain data integrity. This proactive approach minimizes disruption and improves the application’s overall resilience.
Common Error Scenarios and Handling Them Effectively
Numerous error scenarios can arise during event handling. For example, network issues, file access failures, invalid user input, or issues with external services can all lead to exceptions. Effectively handling these scenarios involves employing robust exception handling techniques to identify, catch, and manage these potential problems.
Examples of Graceful Exception Handling during Event Processing
Consider a scenario where an event handler processes data from a file. If the file is not found or is corrupted, an exception will occur. A well-designed handler should catch this exception, log the error, and display a user-friendly message instead of crashing the application. This approach ensures the application remains functional despite the error. Another example involves an event handler that communicates with a remote server.
If the server is unavailable, an exception will be thrown. A robust handler should catch this exception, display a message indicating the server is unavailable, and allow the application to continue without halting. This example highlights the practical application of exception handling in a real-world context.
Code Snippet Demonstrating Error Handling within an Event Handler
“`C#// Example event handler with error handlingprivate void MyEventHandler(object sender, EventArgs e) try // Code that might throw an exception string filePath = “data.txt”; string data = File.ReadAllText(filePath); // Process the data int value = int.Parse(data); Console.WriteLine($”Value: value”); catch (FileNotFoundException ex) Console.WriteLine($”Error: File not found – ex.Message”); // Log the error or take alternative action catch (FormatException ex) Console.WriteLine($”Error: Invalid data format – ex.Message”); // Display an appropriate error message to the user.
catch (Exception ex) Console.WriteLine($”An unexpected error occurred: ex.Message”); // Log the error for debugging purposes “`This code snippet demonstrates a `try-catch` block to handle potential `FileNotFoundException` and `FormatException`. The `catch` blocks provide specific error handling for each exception type, preventing the application from crashing and providing informative error messages.
The `catch` block with `Exception` is a general-purpose handler to catch any unanticipated errors.
Event Handling Best Practices in C# Builder
Salam kenal, fellow developers! Mastering event handling in C# Builder is crucial for building robust and maintainable applications. This section Artikels key best practices to ensure your event handlers are efficient, reliable, and easy to understand. Following these guidelines will lead to cleaner code and a more enjoyable development experience.
Writing Maintainable and Robust Event Handlers
To create maintainable and robust event handlers, meticulous attention to detail is essential. A well-structured approach reduces the likelihood of bugs and simplifies future modifications. This involves clearly defining the scope of each handler, ensuring its functionality is isolated, and avoiding unnecessary complexity.
Always strive for clarity and simplicity in your event handlers. Complex logic should be extracted into separate methods for better organization and maintainability.
Proper Naming Conventions for Events and Handlers
Consistent naming conventions enhance code readability and reduce confusion. Using descriptive names for events and handlers helps convey their purpose.
- Use verbs or verb phrases to describe the action associated with the event. For instance, “OnButtonClicked” or “OnFileLoaded”.
- Use PascalCase for both events and handler names to adhere to C# Builder conventions. This improves code readability and reduces the chance of errors.
- Clearly indicate the object or component triggering the event. For example, “Form1.OnClosing” or “Button1.OnClicked”.
Minimizing Code Duplication and Promoting Code Reusability
Repeated code segments can lead to maintenance headaches and increase the risk of errors. Employing reusable components and functions can minimize code duplication and improve maintainability.
- Identify common event handling tasks and encapsulate them into reusable methods or classes.
- Extract common logic into separate functions, promoting modularity and reusability. This ensures that code is consistent across various parts of the application.
- Create custom event arguments to handle specific data associated with events, reducing code duplication and improving the overall structure.
Handling Events in a Thread-Safe Manner
When dealing with multiple threads, ensuring thread safety is paramount to avoid race conditions and data corruption.
- Employ thread-safe mechanisms to access shared resources when events are triggered by different threads. This includes using locks or synchronization primitives to prevent race conditions.
- Use appropriate locking mechanisms (like mutexes or locks) to protect shared resources from concurrent access when handling events.
- Consider using the `SynchronizingObject` class if you need to ensure thread safety during event handling. This will guarantee consistent and reliable operation, preventing unintended issues.
Error Handling and Event Handling
Proper error handling is vital to ensure application stability. When implementing event handlers, anticipate potential issues and handle them gracefully.
- Include error checks and exception handling to manage potential errors during event handling. This prevents unexpected application behavior and helps in debugging.
- Implement appropriate exception handling mechanisms to catch and manage potential errors. This prevents unexpected crashes and facilitates debugging.
- Use logging or tracing to monitor events and track potential issues. This helps in troubleshooting and identifying the source of problems.
Advanced Event Handling Techniques
Mastering event handling in complex applications often requires more sophisticated techniques than basic event handling. These techniques are crucial for building robust, responsive, and maintainable applications, especially in scenarios involving multiple threads, asynchronous operations, and large event streams. Understanding these advanced methods empowers developers to handle intricate situations with precision and efficiency.
Multithreaded Event Handling
Multithreading introduces unique challenges to event handling. Proper synchronization mechanisms are essential to prevent race conditions and ensure data integrity. When events are handled across multiple threads, careful consideration must be given to thread safety and locking mechanisms to prevent inconsistencies. The use of thread-safe collections and proper synchronization primitives, like locks and mutexes, is vital for maintaining the integrity of shared data.
Asynchronous Event Handling
Asynchronous programming enables applications to perform operations concurrently without blocking the main thread. In event handling, this can improve responsiveness by allowing the application to continue processing other tasks while waiting for an event to complete. Implementing asynchronous event handling typically involves using asynchronous methods, callbacks, or promises to execute event handlers outside the main thread’s execution path.
This approach is particularly valuable when dealing with potentially time-consuming operations triggered by events.
Event Aggregators
Event aggregators provide a centralized mechanism for managing events in large applications. They decouple event publishers from event subscribers, allowing for modularity and flexibility. This approach is highly beneficial in complex applications with many interacting components. Event aggregators act as a mediator, ensuring events reach the intended subscribers without direct coupling between components. This promotes maintainability and reduces the impact of changes in one part of the application on other parts.
Implementing Event Aggregators
Implementing an event aggregator involves several key steps. First, define a base class or interface for events, specifying the properties and data necessary to describe an event. Then, create an event aggregator class responsible for publishing and subscribing to events. Implement methods to publish events and subscribe to events, typically using a dictionary or similar data structure to store event subscriptions.
Crucially, consider error handling in the event aggregator to catch and manage unexpected situations. This proactive approach minimizes the risk of application crashes and ensures smooth operation, even during unexpected events.
Event Type | Description | Example |
---|---|---|
Event Data | Contains details about the event. | UserAction: userId: 123, action: “login” |
Event Aggregator | Centralized event management. | EventAggregator.Publish(new UserAction userId = 123, action = “login”); |
Event Handler | Handles the event. | EventAggregator.Subscribe(e => Console.WriteLine($”User e.userId logged in.”); ); |
A well-designed event aggregator facilitates a clean separation of concerns. This promotes maintainability and reduces the risk of introducing bugs. This approach is highly recommended for applications with numerous components and events, ensuring the application remains responsive and organized.
Conclusion

In conclusion, mastering event handling in C# Builder is key to building dynamic and user-friendly applications. We’ve covered the essential concepts, practical examples, and best practices to help you integrate events seamlessly into your projects. This comprehensive guide has provided a strong foundation for your event handling endeavors.
FAQ Overview: How To Assign Event Handler In C Builder
Q: What are the common types of events used in C# Builder applications?
A: Common event types include button clicks, text box changes, form loads, and mouse movements. You’ll also encounter custom events tailored to your specific application logic.
Q: How do I handle multiple events for a single control?
A: You can handle multiple events using a single event handler, or assign separate handlers for each event type, depending on your needs. Strategies for handling multiple events efficiently are detailed in the guide.
Q: What are delegates, and why are they important for event handling?
A: Delegates act as pointers to methods. In event handling, they allow you to pass methods as arguments, enabling flexible and reusable event handlers.
Q: How can I effectively handle errors within event handlers?
A: Robust event handlers should include error handling to prevent crashes. The guide explains how to gracefully manage exceptions that might arise during event processing.