How to assign event handler in c builder tnotifyeventhandler is your key to unlocking powerful event-driven programming in C# Builder. Learn how to effectively use TNotifyEventHandler to create responsive and dynamic applications. We’ll delve into the intricacies of defining custom events, handling arguments, and assigning handlers, equipping you with the knowledge to build sophisticated and interactive applications.
This comprehensive guide covers everything from basic event handling principles to advanced techniques for working with multiple handlers and specific event types. Clear examples and code snippets will walk you through the process step-by-step, ensuring a deep understanding of the topic. Discover the power of TNotifyEventHandler and build robust, event-driven applications!
Introduction to Event Handling in C# Builder
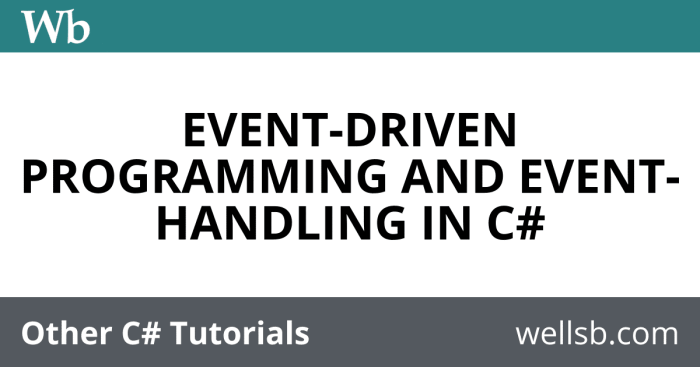
Event handling in C# Builder, a powerful aspect of application development, allows your program to respond dynamically to user actions or internal program changes. This responsiveness is crucial for building interactive and user-friendly applications. It empowers you to create applications that react in real-time to events, making the user experience seamless and intuitive.Event handling, in essence, is a mechanism that enables your application to execute specific code blocks in response to particular events.
This dynamic interaction is a cornerstone of modern application design, providing a flexible framework for constructing applications that react to various triggers, whether from user input or internal program logic.
Role of TNotifyEventHandler
The `TNotifyEventHandler` in C# Builder acts as a delegate type for handling events. A delegate is a reference type that points to a method. This delegate specifically handles notification events, enabling your application to respond to changes in the state of an object. This capability is vital in building applications that maintain a consistent and responsive user interface.
Basic Structure of an Event Handler
An event handler in C# Builder typically involves defining a method that matches the signature of the event delegate (in this case, `TNotifyEventHandler`). This method will contain the code that executes when the event occurs. This structured approach allows for organized and efficient handling of different events within your application. The method signature usually takes an `object sender` and a `TNotifyEventArgs` as parameters, allowing you to identify the source of the event and access any associated data.
Importance of Event Handlers in Application Development
Event handlers are crucial in application development for several reasons. They enable responsiveness to user actions, such as button clicks or form changes. They facilitate communication between different parts of your application. This communication, when handled effectively, enables more robust and sophisticated application behavior. This capability is essential for building complex applications, where different components need to interact and coordinate their actions.
They also enable efficient management of internal application state changes, which leads to a smoother and more efficient application flow.
Simple Example of a Basic Event Handler
This example demonstrates a basic event handler in C# Builder, showing the fundamental structure. It involves a simple event and a handler method that responds to that event.“`C#// Define the eventpublic event TNotifyEventHandler MyEvent;// Method to raise the eventprotected virtual void OnMyEvent(object sender, TNotifyEventArgs e) if (MyEvent != null) MyEvent(sender, e); // Handler methodprivate void MyEventHandler(object sender, TNotifyEventArgs e) // Code to execute when the event occurs MessageBox.Show(“Event triggered!”);// In the constructor or another appropriate placeMyEvent += MyEventHandler;// Raise the eventOnMyEvent(this, new TNotifyEventArgs());“`This concise example highlights the core elements of event handling in C# Builder.
The `OnMyEvent` method is crucial for raising the event, while the `MyEventHandler` method is the event handler. The event is raised by calling `OnMyEvent`, and the handler is invoked when the event is raised. This example illustrates the fundamental principles behind event handling, showing how events are defined, raised, and handled within a C# Builder application. This simplicity allows for efficient and organized handling of various events in your application.
Defining and Using TNotifyEventHandler
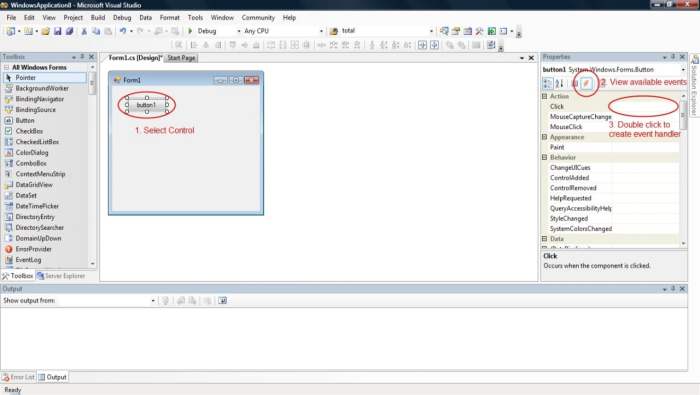
Embarking on the journey of event handling in C++ Builder, understanding how to define and utilize custom events is crucial. This section delves into the specifics of working with `TNotifyEventHandler`, empowering you to create robust and responsive applications. Mastering this technique will significantly enhance your ability to build dynamic and interactive user interfaces.
Defining a Custom Event with TNotifyEventHandler
A custom event, leveraging `TNotifyEventHandler`, allows you to communicate changes or actions between different components within your application. This facilitates a more sophisticated and organized design, enhancing code maintainability and readability. The crucial step involves defining the event’s signature, employing `TNotifyEventHandler`.
Parameters of TNotifyEventHandler
The `TNotifyEventHandler` parameter structure comprises two essential elements: the sender and the event arguments. Understanding their roles is fundamental to effective event handling.
Sender and Event Arguments in TNotifyEventHandler
The `sender` parameter represents the object that raised the event. This provides crucial context, enabling you to determine the source of the notification. The `TEventData` object, part of the event arguments, often contains data relevant to the event. This structured approach allows for a granular and comprehensive notification system.
Example of Custom Event and Handler
This example showcases a `TNotifyEventHandler`-based custom event within a hypothetical scenario. A `TMyComponent` class emits a `OnChange` event, notifying listeners of changes in its internal data.“`C++#include
Declaration of TNotifyEventHandler Event
The following code snippet demonstrates the declaration of an event of type `TNotifyEventHandler`. This is a crucial aspect for defining the structure of your custom event, ensuring that it adheres to the expected format for handling events.“`C++__property TNotifyEvent OnChange;“`This declaration defines a property `OnChange` that represents the custom event. The event is of type `TNotifyEvent`, which is linked to `TNotifyEventHandler`.
Handling Event Arguments
Unlocking the power of event handling in C# Builder often involves passing data between events and their handlers. This crucial aspect, facilitated by event arguments, allows for more sophisticated and adaptable applications. By understanding and effectively utilizing event arguments, you’ll enhance the responsiveness and flexibility of your code. This section will guide you through the nuances of event arguments with TNotifyEventHandler, showcasing their importance and practical application.
Event Arguments in TNotifyEventHandler
Event arguments act as containers for data associated with an event. They provide a structured way to transmit information from the event source to the event handler. In the context of TNotifyEventHandler, these arguments hold details about the event that occurred. This data can include, but is not limited to, the specific property that changed, the new value of the property, or other relevant contextual information.
Common Event Argument Types
Often, event arguments are simple data structures carrying information. For example, a `TNotifyPropertyChangedEventArgs` (used with `TNotifyPropertyChanged`) might contain the name of the property that changed. This allows the handler to know precisely what part of the object has been modified. Similarly, a custom event argument class could contain various properties tailored to the specific needs of your application.
This flexibility is a cornerstone of robust event handling.
Creating Custom Event Arguments
Developing custom event arguments for TNotifyEventHandler allows you to precisely define the information passed to handlers. This tailoring enhances the specific functionality of your events. For instance, if you are building an application dealing with file operations, custom arguments could contain the filename, the operation performed (e.g., “read,” “write”), and the result of the operation. This customized information greatly improves the effectiveness of the event handling mechanism.“`C#// Example of a custom event argument classpublic class FileOperationEventArgs : EventArgs public string FileName get; set; public string Operation get; set; public bool Success get; set; public FileOperationEventArgs(string fileName, string operation, bool success) FileName = fileName; Operation = operation; Success = success; “`This code snippet illustrates a simple `FileOperationEventArgs` class.
It encapsulates the necessary data for a file operation event, enabling your handlers to react to different file operations with more precise information.
Significance of Event Arguments
Event arguments are vital for passing data between events and handlers. Without them, the handler would have limited access to the event’s context. This lack of context leads to more cumbersome and less efficient code. Event arguments ensure that handlers receive the necessary information to respond appropriately to the event. They are fundamental to building dynamic and data-driven applications.
Example Using Event Arguments
Consider a scenario where a form updates its UI elements based on file operations. A `FileOperationEventArgs` object is used to transmit information about the file operation.“`C#// … (previous code)// Example of raising the eventprivate void PerformFileOperation(string fileName, string operation) bool success = true; // Example: Replace with actual operation result FileOperationEventArgs args = new FileOperationEventArgs(fileName, operation, success); FileOperation?.Invoke(this, args);// Example of a handlerprivate void FileOperation_EventHandler(object sender, FileOperationEventArgs e) if (e.Success) // Update UI to reflect successful file operation MessageBox.Show($”File e.FileName e.Operation successfully.”); else // Handle failure MessageBox.Show($”Error processing file e.FileName.”); “`This comprehensive example demonstrates how to raise a custom event, `FileOperation`, and provide event arguments to the handler.
The handler, in turn, utilizes the information in the arguments to respond appropriately. This illustrates the power and practicality of using event arguments for precise and controlled event handling.
Assigning Event Handlers in C# Builder
Unlocking the power of event handling in C# Builder empowers you to create dynamic and responsive applications. Event handlers are crucial for connecting user actions or internal program changes to specific code responses. This process, though straightforward, is a fundamental building block in developing interactive software. Mastering this technique will greatly enhance your programming skills and your ability to craft applications that react intelligently to user input and internal events.
Connecting a Method to a TNotifyEventHandler Event
Successfully connecting a method to a TNotifyEventHandler event is a key step in building interactive applications. This process allows your application to respond to specific events by executing pre-defined code. This involves defining a method that matches the event’s signature and then registering that method as the handler for the event. This creates a direct link between the event occurrence and the execution of the designated method.
Steps for Assigning a Handler
- Identify the Event: Determine the specific event you wish to handle. This is crucial for targeting the correct event source.
- Define the Handler Method: Create a method that conforms to the TNotifyEventHandler signature. This method will be executed when the event occurs.
- Connect the Handler: Use the `Add` method of the event to register your handler method with the event. This crucial step establishes the connection between the event and the handler.
Detailed Code Example
This example demonstrates assigning a handler to a `TNotifyEventHandler` event. The `OnNotify` method will be triggered whenever the `Notify` event is raised.“`C#using System;public class MyClass public event TNotifyEventHandler Notify; public void RaiseNotify() if (Notify != null) Notify(this, EventArgs.Empty); public void MyMethod(object sender, EventArgs e) Console.WriteLine(“Event handled!”); public delegate void TNotifyEventHandler(object sender, EventArgs e);public class Program public static void Main(string[] args) MyClass myObject = new MyClass(); // Connect the handler method myObject.Notify += myObject.MyMethod; // Raise the event myObject.RaiseNotify(); “`This code demonstrates a simple example.
In a real-world application, `MyMethod` would contain the specific code to be executed when the `Notify` event occurs.
Multiple Handler Assignment Examples
You can assign multiple handlers to the same event. Each handler will be executed in the order they are registered.“`C#// … (MyClass and RaiseNotify from previous example) …public class Program public static void Main(string[] args) MyClass myObject = new MyClass(); myObject.Notify += myObject.MyMethod; myObject.Notify += AnotherMethod; // Adding another handler // …
(RaiseNotify) … static void AnotherMethod(object sender, EventArgs e) Console.WriteLine(“Another method handled the event!”); “`This enhanced example illustrates how to add another handler method (`AnotherMethod`) to the same event.
Potential Errors
- Incorrect Handler Signature: Ensuring the handler method precisely matches the TNotifyEventHandler signature is critical. A mismatch will result in compilation errors or unexpected behavior.
- Null Reference Exception: If the event is null, attempting to raise the event will trigger a null reference exception. Always check if the event is not null before raising it.
Working with Multiple Event Handlers
Embarking on the journey of handling multiple event handlers for a single event in C# Builder opens doors to sophisticated applications. This approach empowers you to create dynamic and responsive systems, enabling the seamless integration of diverse functionalities. Multiple event handlers allow for a flexible and scalable approach to managing events, facilitating a robust and maintainable codebase.Multiple event handlers, a powerful feature in C# Builder, provide a mechanism to execute different actions in response to a single event.
This flexibility enhances the application’s ability to react in various ways, adapting to different conditions and scenarios. This versatility is a cornerstone of creating robust and adaptable software systems.
Adding Event Handlers
Adding multiple event handlers for a single event involves attaching multiple event-handling methods to the event. This process allows different parts of your application to respond to the same event in their own unique way. The process is straightforward, similar to assigning a single handler. Each handler is executed in sequence, and the application’s behavior is determined by the cumulative effect of these responses.
Removing Event Handlers
Removing event handlers is equally crucial for managing the responsiveness of your application. This process ensures that specific handlers are no longer triggered when the event occurs, preventing unintended actions or conflicts. Carefully removing handlers is essential for maintaining a clean and efficient event handling system.
Event Delegation and Multiple Handlers
The concept of event delegation plays a vital role in handling multiple event handlers. Event delegation is a mechanism that allows a single event handler to act as a central point for coordinating the execution of multiple handlers. This central handler dispatches the event to the various registered handlers, enabling a more organized and controlled response. This paradigm allows for cleaner and more maintainable code.
Example: Handling Multiple Event Handlers
This example demonstrates the assignment of multiple event handlers to a TNotifyEvent:“`C#unit Unit1;interfaceuses Winapi.Windows, Winapi.Messages, System.SysUtils, System.Variants, System.Classes, Vcl.Controls, Vcl.Forms, Vcl.Dialogs;type TForm1 = class(TForm) Button1: TButton; procedure Button1Click(Sender: TObject); private Private declarations public Public declarations end;var Form1: TForm1;implementation$R – .dfmprocedure TForm1.Button1Click(Sender: TObject);begin // Example of raising an event if Assigned(NotifyEvent) then NotifyEvent.OnNotify(Self, ‘Button Clicked’);end;// Example of an eventtype TNotifyEvent = class(TObject) public procedure OnNotify(Sender: TObject; Note: string); published property OnNotify: TNotifyEvent; end;procedure TForm1.TNotifyEvent.OnNotify(Sender: TObject; Note: string);var i: integer;begin for i := 0 to Length(Handlers) – 1 do Handlers[i](Sender, Note);end;var NotifyEvent: TNotifyEvent; Handlers: array of TNotifyEventHandler;procedure AddHandler(Handler: TNotifyEventHandler);begin SetLength(Handlers, Length(Handlers) + 1); Handlers[Length(Handlers)
1]
= Handler;end;procedure RemoveHandler(Handler: TNotifyEventHandler);var i: Integer;begin for i := 0 to Length(Handlers) – 1 do if Handlers[i] = Handler then begin SetLength(Handlers, Length(Handlers) – 1); Exit; end;end;// Example of attaching event handlersprocedure TForm1.FormCreate(Sender: TObject);var Handler1, Handler2: TNotifyEventHandler;begin NotifyEvent := TNotifyEvent.Create; Handler1 := procedure(Sender: TObject; Note: string) begin ShowMessage(‘Handler 1: ‘ + Note); end; Handler2 := procedure(Sender: TObject; Note: string) begin ShowMessage(‘Handler 2: ‘ + Note); end; AddHandler(Handler1); AddHandler(Handler2);end;end.“`This structured example illustrates the addition of handlers.
The `AddHandler` procedure dynamically adds handlers to the event. The `RemoveHandler` procedure efficiently removes handlers from the event. This demonstrates the power and flexibility of managing multiple handlers in C# Builder.
Handling Specific Event Types with TNotifyEventHandler
Unlocking the power of event handling in C# Builder often involves discerning specific event types. TNotifyEventHandler, while a versatile tool, can be further enhanced by understanding how to tailor your event handling to the nuances of particular events. This approach empowers you to react effectively to diverse scenarios and extract valuable data from each event occurrence.Event types act as crucial identifiers, distinguishing one event from another.
Knowing which event type is occurring allows you to perform specific actions based on the event’s nature. Consider this as a sophisticated filtering system, enabling targeted responses to distinct situations. This meticulous approach ensures your application’s robustness and responsiveness.
Event Type Identification
By meticulously inspecting the event type, you gain the ability to react selectively. This capability is pivotal in intricate applications where various events demand tailored responses. The event type essentially dictates the course of action your code should take.
Examples of Event Handling with Specific Event Types
Consider an application where a user can perform various actions, such as adding, removing, or updating items in a list. Each action triggers a distinct event, and your code can respond accordingly.
- Adding an Item: When a user adds an item to the list, the application triggers an “ItemAdded” event. Your event handler, using TNotifyEventHandler, can be designed to capture the details of the added item, such as its name, value, and position in the list. This allows you to perform operations such as updating the display or saving the changes to a database.
This demonstrates a clear delineation of the event handling for a specific type of action.
- Removing an Item: Similarly, removing an item triggers an “ItemRemoved” event. The handler, with TNotifyEventHandler, can capture the removed item’s details and update the application’s state accordingly, for instance, removing the corresponding record from a database or refreshing the display.
- Updating an Item: Updating an item results in an “ItemUpdated” event. This event type informs the application about the change and enables your event handler, using TNotifyEventHandler, to retrieve the updated item details and apply the modifications to the underlying data structure or database.
Utilizing Event Arguments for Specific Data
Event arguments are indispensable components of event handling. They encapsulate the data specific to the event that occurred. By accessing this data, your event handlers can react appropriately. This data-rich approach is essential for applications that require detailed responses to complex events.
- Detailed Event Data: Each event type, like “ItemAdded,” carries an event argument. This argument might include properties such as the item’s name, value, and index in the list. This comprehensive information is vital for targeted actions within your event handler.
Importance of Event Types in Distinguishing Scenarios
Event types are crucial for differentiating between different events. Without them, you might be unable to distinguish between an item being added, removed, or updated. This clear distinction is vital for complex applications with many possible user interactions. Without distinct event types, the application might react inappropriately or fail to respond correctly to specific situations.
Limitations of TNotifyEventHandler with Complex Events
While TNotifyEventHandler is powerful, it has limitations when handling events with intricate structures. For example, if an event involves multiple complex data objects or intricate relationships, TNotifyEventHandler might prove insufficient. More specialized event handling mechanisms might be necessary for managing these complex events effectively.
Best Practices and Considerations
Mastering event handling in C# Builder using `TNotifyEventHandler` unlocks powerful responsiveness in your applications. This section delves into best practices, potential pitfalls, and various approaches to ensure efficient and robust event handling. Understanding these nuances empowers you to write cleaner, more maintainable, and ultimately, more effective C# Builder code.
Best Practices for Assigning Event Handlers
Effective event handling hinges on meticulous assignment. Prioritize using strongly-typed delegates whenever possible. This improves code readability and helps catch potential type mismatches at compile time. Always ensure the event handler method signature aligns precisely with the `TNotifyEventHandler` delegate’s expected parameters. This prevents runtime errors and enhances code reliability.
Avoid assigning event handlers within the constructor, unless absolutely necessary. Instead, handle events in a separate method or within an event-driven component for better organization and maintainability.
Potential Pitfalls and Common Errors
One frequent pitfall involves forgetting to unsubscribe from events when they are no longer needed. This can lead to memory leaks or unexpected behavior. Always unsubscribe from events when the object or component handling them is no longer required. Another common error involves handling events in an overly complex or tightly coupled manner. Decompose event handling logic into smaller, more manageable methods to enhance maintainability and readability.
Ensure that event handlers do not modify the object’s state directly, unless absolutely necessary. This improves testability and prevents unexpected side effects.
Comparing Different Approaches to Event Handling
Various strategies exist for handling events. While `TNotifyEventHandler` is well-suited for simple notification events, consider using more sophisticated approaches for complex scenarios. For instance, event aggregators can provide a more flexible and decoupled way to handle events in large applications, facilitating communication between different parts of the application. Weigh the complexity of the application and the nature of the events when choosing an event handling strategy.
Situations Where TNotifyEventHandler is Most Suitable
`TNotifyEventHandler` excels in scenarios requiring simple notifications about property changes. Think of scenarios where a control needs to inform its parent about a specific state update, or where a component needs to react to a data change in a model. Use `TNotifyEventHandler` when the event’s purpose is primarily informational, and the event handler simply needs to perform a specific action or update the UI in response.
This is often found in data binding and UI synchronization tasks.
Summary of Important Points, How to assign event handler in c builder tnotifyeventhandler
To effectively use `TNotifyEventHandler`, remember these key points:
- Employ strongly-typed delegates for improved code reliability.
- Ensure event handler signatures match `TNotifyEventHandler`’s parameters.
- Unsubscribing from events is crucial to prevent memory leaks.
- Keep event handlers concise and maintainable, breaking down complex logic.
- Prioritize informational events and avoid direct state modifications.
Illustrative Examples and Code Snippets
Unlocking the power of event handling in C++ Builder with TNotifyEventHandler is simplified by practical examples. These examples will illuminate the process, making event handling intuitive and empowering you to build robust applications.Mastering event handling is crucial for creating interactive and responsive applications. Clear code snippets, detailed explanations, and comprehensive examples will guide you through the intricacies of this essential programming concept.
Form with Button and Text Box
This example demonstrates event handling in a form with a button and a text box. Clicking the button updates the text box content. This illustrates a fundamental event-driven paradigm.“`C++#include
Form1 = new TMyForm(Application);
Application->Run(); delete Form1; return 0;“`This code snippet showcases the `Button1Click` event handler. When the button is clicked, the `Edit1->Text` property is updated with a message. This is a clear demonstration of how to assign and handle events in a form.
Custom Class with Events
Demonstrating event handling within a custom class. This example showcases a class that raises events when a specific condition is met.“`C++#include
Sender, const string& message)
TMyClass* myClass = static_cast
Common Scenarios
A collection of code snippets showcasing common scenarios in event handling.
- Handling button clicks: The previous examples demonstrate how to handle button clicks. This is a basic but essential application of events.
- Responding to form resizing: The form resize event can trigger updates or actions based on the new dimensions of the form. This is a typical way to maintain visual elements in the correct proportions.
- Data validation: A validation event could occur before saving data, allowing for checks on input correctness. This example ensures data quality.
- Custom controls: Custom controls can raise events to signal specific conditions or actions within the control itself. This allows for a more granular control of functionality.
Comprehensive Component Example
This example showcases a component that raises events of type TNotifyEventHandler. This example shows how a component can react to external events and communicate with other parts of the application.“`C++// … (component header)class TMyComponent : public TComponent // … (other members) TNotifyEvent OnMyEvent; void RaiseMyEvent(string message) if (OnMyEvent != nullptr) OnMyEvent(this, message); ;// …
(component implementation)void __fastcall TMyComponent::RaiseMyEvent(string message) if (OnMyEvent != nullptr) OnMyEvent(this, message);// … (other component methods)// … (Usage example)int main() // … TMyComponent* myComponent = new TMyComponent(); myComponent->OnMyEvent = MyEventHandler; // Assign the handler myComponent->RaiseMyEvent(“Component Event!”); // …“`This example effectively demonstrates how a custom component can be designed to raise and handle events, providing a more modular and reusable design structure.
Advanced Topics (Optional): How To Assign Event Handler In C Builder Tnotifyeventhandler
Unlocking the full potential of event handling in C# Builder involves delving into advanced techniques. This section explores sophisticated approaches, including asynchronous operations, delegate integration, multithreading considerations, custom event types, and complex scenario management. These advanced techniques empower developers to build robust and responsive applications capable of handling intricate tasks efficiently.Mastering these advanced concepts allows you to create applications that are not only functional but also performant and adaptable to various demands.
Event handling becomes a powerful tool, enabling your applications to respond to dynamic situations with grace and efficiency.
Asynchronous Operations in Event Handling
Event handling often involves operations that take time to complete. Implementing asynchronous operations within event handlers ensures your application remains responsive during these processes. This approach prevents the application from freezing while waiting for long-running tasks to finish. By utilizing asynchronous methods and tasks, your application can maintain a smooth user experience, even when dealing with time-consuming operations.
Delegates and TNotifyEventHandler
Delegates are powerful tools that allow you to encapsulate methods and pass them as arguments. This enables flexible and dynamic event handling. By combining delegates with `TNotifyEventHandler`, you can create highly adaptable and reusable event handling mechanisms. This flexible approach enhances code modularity and reduces code duplication, leading to a cleaner and more maintainable application.
Event Handling in Multithreaded Environments
Event handling in multithreaded environments necessitates careful consideration of thread safety. Ensuring thread safety prevents data corruption and application crashes. By employing appropriate synchronization mechanisms, such as locks and mutexes, your application can effectively manage concurrent access to shared resources and ensure data integrity.
Creating Custom Event Types with TNotifyEventHandler
Custom event types are essential for extending event handling beyond the built-in capabilities. By creating custom event types, you can tailor the events to your application’s specific needs, encapsulating the relevant data and behavior. This approach allows for greater flexibility and control over the events within your application. Creating custom events allows you to manage complex interactions with precisely defined data structures.
Handling Event Handling in Complex Scenarios
Event handling in complex scenarios often involves chains of events and intricate interactions between different components. Managing these intricate interactions effectively requires careful planning and a well-structured design. This approach ensures that your application responds appropriately to complex and interconnected events, facilitating a smooth and predictable execution flow. By carefully defining the sequence and dependencies of events, you create a robust application capable of handling multifaceted interactions.
Conclusion
Mastering event handling with TNotifyEventHandler in C# Builder empowers you to create sophisticated applications. This guide has provided a solid foundation, from the fundamentals to advanced techniques. Remember the key concepts and best practices for optimal results. Now go forth and build remarkable applications!
FAQ
What’s the difference between TNotifyEventHandler and other event handler types in C# Builder?
TNotifyEventHandler is specifically designed for handling notifications of property changes. Other event handlers might be used for different types of events.
How can I debug issues with event handler assignments?
Use breakpoints in your debugger to inspect the values of sender and event arguments. Check for null references and ensure correct method signatures.
What are common pitfalls when working with multiple event handlers?
Ensure proper handling of adding and removing handlers, especially in multithreaded environments. Incorrect removal can lead to memory leaks or unexpected behavior.
Can I use TNotifyEventHandler for asynchronous operations?
While possible, asynchronous operations with TNotifyEventHandler require careful consideration. Consider using asynchronous delegates or tasks for better management of concurrency.