How to remove node fom nested json object with array.filter unveils a profound journey into the intricate world of data manipulation. This exploration delves into the art of pruning nested JSON structures, revealing the hidden elegance within. We’ll uncover the secrets of array.filter, a powerful tool for meticulously removing specific nodes while preserving the integrity of the remaining data.
Prepare to embark on a path of enlightenment, where the intricate dance of data transforms into a harmonious symphony of efficiency.
Nested JSON objects, with their complex arrays, often present a challenge to the aspiring data wrangler. Understanding how to navigate these intricate structures is key to mastering the art of data manipulation. This exploration provides a clear and concise guide to removing nodes from nested JSON objects using the array.filter method, empowering you to transform raw data into a refined masterpiece.
We will guide you through the process step-by-step, illustrating each concept with practical examples and illustrative tables.
Introduction to Nested JSON Objects and Arrays
JSON (JavaScript Object Notation) is a lightweight data-interchange format. It’s often used to transmit data between a server and a web application. Nested JSON objects and arrays allow for representing complex data structures, enabling the storage and retrieval of intricate relationships between data points. Understanding these structures is crucial for efficiently working with JSON data.Nested JSON objects and arrays enable the representation of hierarchical data, where one data structure is contained within another.
This hierarchical structure mirrors real-world relationships and allows for the storage of detailed information within a single data block. It’s a powerful tool for managing and organizing large amounts of information.
Structure of Nested JSON Objects and Arrays
JSON objects are key-value pairs enclosed in curly braces “, while arrays are ordered lists of values enclosed in square brackets `[]`. A nested structure combines these elements, where objects can contain arrays, and arrays can contain objects or other data types.
Accessing Elements Within Nested Structures
Accessing elements within nested JSON structures involves using dot notation or bracket notation for objects and array indexes for arrays. This method allows for precise location and retrieval of specific data points.
Example of a Nested JSON Object with an Array
This example demonstrates a nested structure:“`json “name”: “John Doe”, “age”: 30, “courses”: [ “title”: “Introduction to Programming”, “credits”: 3, “title”: “Data Structures and Algorithms”, “credits”: 4 ]“`
Table Illustrating the Structure
This table Artikels the structure of the example JSON object:
Key | Data Type | Description |
---|---|---|
name | String | Student’s name |
age | Integer | Student’s age |
courses | Array | List of courses taken |
title | String | Course title |
credits | Integer | Number of credits for the course |
Understanding the Problem: Removing Nodes
Removing a node from a nested JSON object containing an array requires careful consideration of the data structure and potential consequences. Incorrect removal can lead to data corruption and inconsistencies within the overall dataset. Understanding the specific scenarios where removal is necessary and the potential challenges is crucial to ensure data integrity and maintain the structure of the remaining data.Removing nodes from nested JSON objects, especially those containing arrays, is a common operation in data manipulation and processing.
It’s essential to approach this with precision to avoid unintended side effects on the integrity of the data. The process involves not only identifying the node to be removed but also ensuring the surrounding structure remains valid and consistent. Careful planning and consideration of various scenarios are vital to avoid data loss or errors.
Scenarios for Node Removal
The necessity for removing nodes in nested JSON objects arises in various situations. For example, outdated or irrelevant data might need to be purged. Data that violates specific criteria or does not meet required standards can also be removed. This can be a part of data cleaning or validation processes.
Potential Challenges in Removal
Several challenges can arise during the removal process. One challenge is ensuring that the removal operation doesn’t unintentionally affect other parts of the data structure. Another challenge is handling complex nested structures, where the node to be removed might be deeply embedded within the object. The complexity of the nested structure directly affects the level of care and attention required during the removal process.
The potential for errors in handling deeply nested structures increases significantly. Maintaining data integrity and preventing unintended side effects during the removal process are paramount.
Preserving Structure
Preserving the structure of the remaining data is crucial. Changes to the structure could have unintended consequences on subsequent data processing or analysis. A meticulous approach is needed to avoid disruptions to the data’s overall coherence.
Example JSON Object
Consider the following JSON object:“`json “products”: [ “id”: 1, “name”: “Product A”, “category”: “Electronics”, “id”: 2, “name”: “Product B”, “category”: “Clothing”, “id”: 3, “name”: “Product C”, “category”: “Electronics” ], “settings”: “theme”: “dark”, “language”: “English” “`This example demonstrates a nested JSON object with an array of products and a settings object.
The goal is to remove the product with `id` 2.
Expected Outcome
The expected outcome after the removal operation is a new JSON object with the product with `id` 2 removed.
Removal Operation
Before Removal | After Removal |
---|---|
```json "products": [ "id": 1, "name": "Product A", "category": "Electronics", "id": 2, "name": "Product B", "category": "Clothing", "id": 3, "name": "Product C", "category": "Electronics" ], "settings": "theme": "dark", "language": "English" ``` |
```json "products": [ "id": 1, "name": "Product A", "category": "Electronics", "id": 3, "name": "Product C", "category": "Electronics" ], "settings": "theme": "dark", "language": "English" ``` |
Methods for Removal: How To Remove Node Fom Nested Json Object With Array.filter
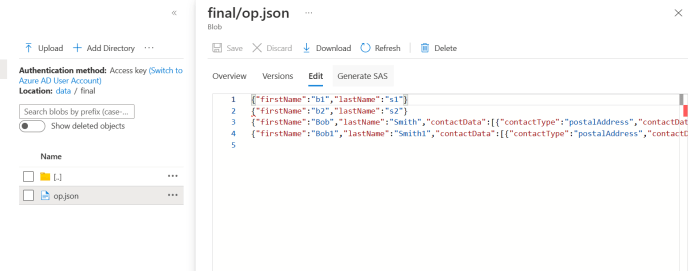
In the realm of data manipulation, efficiently removing specific elements from nested JSON objects and arrays is a crucial skill. This process is essential for maintaining data integrity and ensuring the accuracy of analyses. Understanding various approaches and their implications is paramount for effective data management.
Removing nodes from nested JSON objects necessitates careful consideration of the structure and the desired outcome. The method chosen must be tailored to the specific structure of the JSON data and the criteria for removal. Incorrectly implemented methods can lead to unintended consequences, such as data loss or corruption.
Array.filter Method
The `array.filter()` method in JavaScript is a powerful tool for selecting elements from an array based on a specified condition. It creates a new array containing only the elements that pass the test implemented by the provided function. This method preserves the original array, unlike methods like `splice()`, which modify the original array directly.
Implementing Array.filter for Removal
To remove specific elements from an array within a nested JSON object using `array.filter()`, you must define a function that acts as a filter. This function should evaluate each element and return `true` if it should be included in the new array and `false` otherwise. This new filtered array will then replace the original array within the nested JSON object.
Step-by-Step Procedure, How to remove node fom nested json object with array.filter
- Identify the specific nested array within the JSON object that needs modification.
- Define a function that takes an element from the array as input and returns a boolean value based on the criteria for removal (e.g., whether the element’s value matches a certain string).
- Apply the `array.filter()` method to the target array, passing the defined function as an argument.
- Update the original JSON object, replacing the filtered array with the new array returned by `array.filter()`.
Example
Consider the following JSON object:
“`javascript
let jsonData =
“data”: [
“name”: “Apple”, “price”: 1,
“name”: “Banana”, “price”: 0.5,
“name”: “Orange”, “price”: 0.75
]
;
“`
To remove the element where the price is 0.5, use the following code:
“`javascript
let filteredData = jsonData.data.filter(item => item.price !== 0.5);
jsonData.data = filteredData;
“`
This code will result in `jsonData.data` now containing only the elements where price is not 0.5.
Comparison of Approaches
| Method | Advantages | Disadvantages |
|—————–|——————————————————————————————————-|——————————————————————————————————————–|
| `array.filter()` | Creates a new array, preserving the original array.
Easy to understand and implement. Flexible for various filtering criteria. | Requires defining a filtering function, potentially making it less concise for simple removals. |
| `array.splice()` | Modifies the original array directly, potentially more efficient for in-place modifications. | Can be less readable and more error-prone if not carefully implemented, as it directly modifies the original.
|
Alternative Methods
While `array.filter()` is a common and effective approach, other methods like `array.forEach()` combined with a temporary array can also achieve the same result. However, `array.filter()` generally provides a more concise and readable solution for filtering elements.
Illustrative Examples and Scenarios
Understanding JSON objects with arrays, and how to effectively remove nodes, is crucial for efficient data manipulation. This section provides practical examples demonstrating various removal scenarios, from simple to complex, and includes considerations for edge cases. Proper handling of these scenarios ensures data integrity and prevents unexpected errors.
Simple JSON Removal
This example demonstrates the removal of a single element from a simple JSON array. We will use the `filter` method to achieve this.
Original JSON | Removed Element | Resulting JSON |
---|---|---|
“`json “data”: [1, 2, 3, 4, 5] “` |
The element 3 |
“`json “data”: [1, 2, 4, 5] “` |
The `filter` method creates a new array, leaving the original array unchanged. This new array contains elements that satisfy the specified condition. In this case, the condition is to exclude the number 3.
Nested JSON Removal
This example showcases the removal of a node within a deeply nested JSON structure.
Original JSON | Removed Element | Resulting JSON |
---|---|---|
“`json “data”: [“level1”: 1, “level2”: [“level3”: 3, “level4”: 4], “level1”: 2] “` |
The node with level3: 3 |
“`json “data”: [“level1”: 1, “level2”: [ ], “level1”: 2] “` |
The removal of the nested node is accomplished by filtering through each level of the JSON structure until the target element is found. Carefully crafted conditions ensure accurate targeting and avoid unintended consequences.
Removing Elements Based on Criteria
This example demonstrates the removal of elements based on a specific condition, such as a numerical value or index.
Original JSON | Condition | Resulting JSON |
---|---|---|
“`json “data”: [“id”: 1, “value”: “A”, “id”: 2, “value”: “B”, “id”: 3, “value”: “C”] “` |
Removing elements with id=2 |
“`json “data”: [“id”: 1, “value”: “A”, “id”: 3, “value”: “C”] “` |
The `filter` method, combined with appropriate conditional logic, enables targeted removal based on the specified criteria.
Updating the JSON Object
Updating the JSON object after removal involves creating a new object with the filtered data. Avoid directly modifying the original JSON to preserve its integrity.
Original JSON | Action | Resulting JSON |
---|---|---|
“`json “data”: [“id”: 1, “value”: “A”, “id”: 2, “value”: “B”] “` |
Removing element with id = 2 |
“`json “data”: [“id”: 1, “value”: “A”] “` |
This process is essential for maintaining the integrity and reliability of the data structure.
Error Handling and Validation

Ensuring the integrity of data during removal from nested JSON objects is crucial. Errors can arise from various sources, including incorrect data types, missing keys, or malformed JSON structures. Robust error handling is essential to prevent unexpected program crashes and maintain data consistency. Proper validation before attempting removal safeguards against these issues.
Potential Errors
The process of removing nodes from nested JSON objects can encounter several errors. These include issues with the structure of the JSON data, the presence of missing keys, incorrect data types, and invalid input parameters. The presence of invalid JSON or data that does not conform to the expected structure or format can lead to unpredictable results or even program crashes.
A failure to validate input data can cause a program to misinterpret the structure and cause errors during removal.
Preventing and Handling Errors
Preventing errors begins with thorough validation of the input data. This involves checking the structure of the JSON, confirming the presence of expected keys, and verifying data types. Appropriate error handling mechanisms are vital for gracefully handling potential problems. These mechanisms can include using `try…except` blocks in programming languages to catch exceptions and provide informative error messages.
This approach ensures that the program continues to run even if errors occur, preventing unexpected crashes and maintaining data integrity.
Illustrative Examples
Consider the following example with a Python script demonstrating error handling for removing a node:
“`python
import json
def remove_node(data, key):
try:
if not isinstance(data, dict):
raise TypeError(“Input data must be a dictionary.”)
if key not in data:
raise KeyError(f”Key ‘key’ not found in the data.”)
if isinstance(data[key], list):
data[key] = [item for item in data[key] if item != ‘remove’]
elif isinstance(data[key], dict):
data[key] = # or handle the removal of nested dict.
return data
except (TypeError, KeyError) as e:
print(f”Error: e”)
return None # or raise the exception to be handled at a higher level
data =
“name”: “John Doe”,
“age”: 30,
“address”: “street”: “123 Main St”, “city”: “Anytown”,
“hobbies”: [“reading”, “hiking”, “remove”]
result = remove_node(data, “hobbies”)
if result:
print(json.dumps(result, indent=4))
“`
This code demonstrates checking for the correct data type and the presence of the key. It also handles lists and nested dictionaries.
Validation Best Practices
Validating the data before removal is paramount. Validate the JSON structure against a schema to ensure it conforms to expected formats. Use appropriate data type checks to verify the correctness of data values. For example, check if a field containing an age is an integer or if a field for a name is a string. These checks prevent unexpected behavior and maintain data consistency.
Data Inconsistencies
Removing nodes from nested JSON objects can lead to data inconsistencies if the removal is not handled carefully. Removing a key from a nested dictionary that is referenced elsewhere in the data can cause errors. The removal of items from an array might leave gaps or alter the intended structure of the data. It’s important to thoroughly consider the impact of removal on other parts of the data.
Error Handling Table
Potential Error | Description | Solution |
---|---|---|
Incorrect Data Type | Input data is not a dictionary or list. | Use `isinstance()` to check the type before proceeding. Raise a `TypeError` with a descriptive message. |
Key Not Found | The key to be removed does not exist in the JSON object. | Use `in` operator to check for the key. Raise a `KeyError` with the specific key. |
Invalid JSON Format | The input JSON is not well-formed. | Use a JSON parser (e.g., `json.loads()` in Python) to validate the JSON structure. Catch `json.JSONDecodeError`. |
Nested Structure Issues | The key to be removed is part of a nested structure that needs special handling. | Check for nested lists and dictionaries. Use recursive functions to handle nested structures properly. |
Code Examples (JavaScript)
These examples demonstrate removing nodes from nested JSON objects using JavaScript’s `array.filter` method. Proper handling of different data types and error conditions is crucial for robust applications. Understanding these techniques strengthens our ability to manipulate and process complex data structures.
JavaScript Code Example: Removing a Specific Node
This example focuses on removing a specific object from a nested array within a JSON object.
function removeNode(jsonData, targetId) if (typeof jsonData !== 'object' || jsonData === null) return "Invalid JSON data"; const updatedData = JSON.parse(JSON.stringify(jsonData)); // Important: Create a copy if (Array.isArray(updatedData.nestedArray)) updatedData.nestedArray = updatedData.nestedArray.filter(item => item.id !== targetId); else return "nestedArray not found or not an array"; return JSON.stringify(updatedData, null, 2); const jsonData = "nestedArray": [ "id": 1, "name": "Apple", "id": 2, "name": "Banana", "id": 3, "name": "Orange" ] ; const targetIdToRemove = 2; const updatedJson = removeNode(jsonData, targetIdToRemove); if (typeof updatedJson === 'string' && updatedJson.startsWith('') && updatedJson.endsWith('')) console.log(updatedJson); else console.error("Error:", updatedJson);
The function removeNode
takes the JSON data and the target ID as input. It first checks if the input data is a valid JSON object. Crucially, it creates a deep copy of the original data using JSON.parse(JSON.stringify(jsonData))
to avoid modifying the original object. This prevents unintended side effects. It then filters the nestedArray
, keeping only items where the id
does not match the targetId
.
Finally, it converts the modified data back to a string and returns it. Error handling is included to address cases where the input is not valid JSON or the `nestedArray` is not found.
Handling Different Data Types
The example above illustrates handling a specific case where we need to remove an object from a nested array. To remove items with other data types or nested structures, adapt the filter
criteria within the function.
// Example for removing objects with a specific name const jsonData2 = nestedArray: [ id: 1, name: "Apple", id: 2, name: "Banana", id: 3, name: "Orange", ] ; const updatedJson2 = removeNode(jsonData2, "Banana"); // Change to string for name console.log(updatedJson2);
This demonstrates a case where you might need to filter based on a different property, like ‘name’ instead of ‘id’. Modify the filter condition in the removeNode
function accordingly.
Closing Summary
In conclusion, mastering the technique of removing nodes from nested JSON objects using array.filter empowers us to sculpt and refine data with precision. The methods explored in this guide not only offer solutions but also illuminate the underlying principles of data manipulation. Through careful consideration of structure, and the application of the array.filter method, we unlock the full potential of our data, transforming raw information into insightful knowledge.
The insights gained will prove invaluable in countless applications, enhancing our ability to work with and interpret complex data structures.
Questions Often Asked
What are some common error types when removing nodes from nested JSON objects?
Common errors include incorrect indexing, missing elements, and type mismatches. Thorough validation and error handling are crucial to prevent unexpected outcomes.
How do I handle deeply nested JSON structures?
Recursive functions are often necessary for traversing deeply nested structures. Carefully consider base cases and termination conditions to avoid infinite loops.
Can array.filter be used to remove nodes based on multiple criteria?
Yes, by combining multiple filter conditions, such as using logical operators (and/or), you can tailor the removal process to specific requirements. For example, you can filter by value and index simultaneously.
What are the performance implications of using array.filter for node removal?
Array.filter, when implemented correctly, is generally efficient for node removal. However, the performance depends on the size of the array and the complexity of the filtering criteria. Avoid unnecessary iterations to maintain optimal performance.